Threefold Grid v0.8.1 published on Wednesday, Nov 6, 2024 by Threefold
threefold.Network
Explore with Pulumi AI
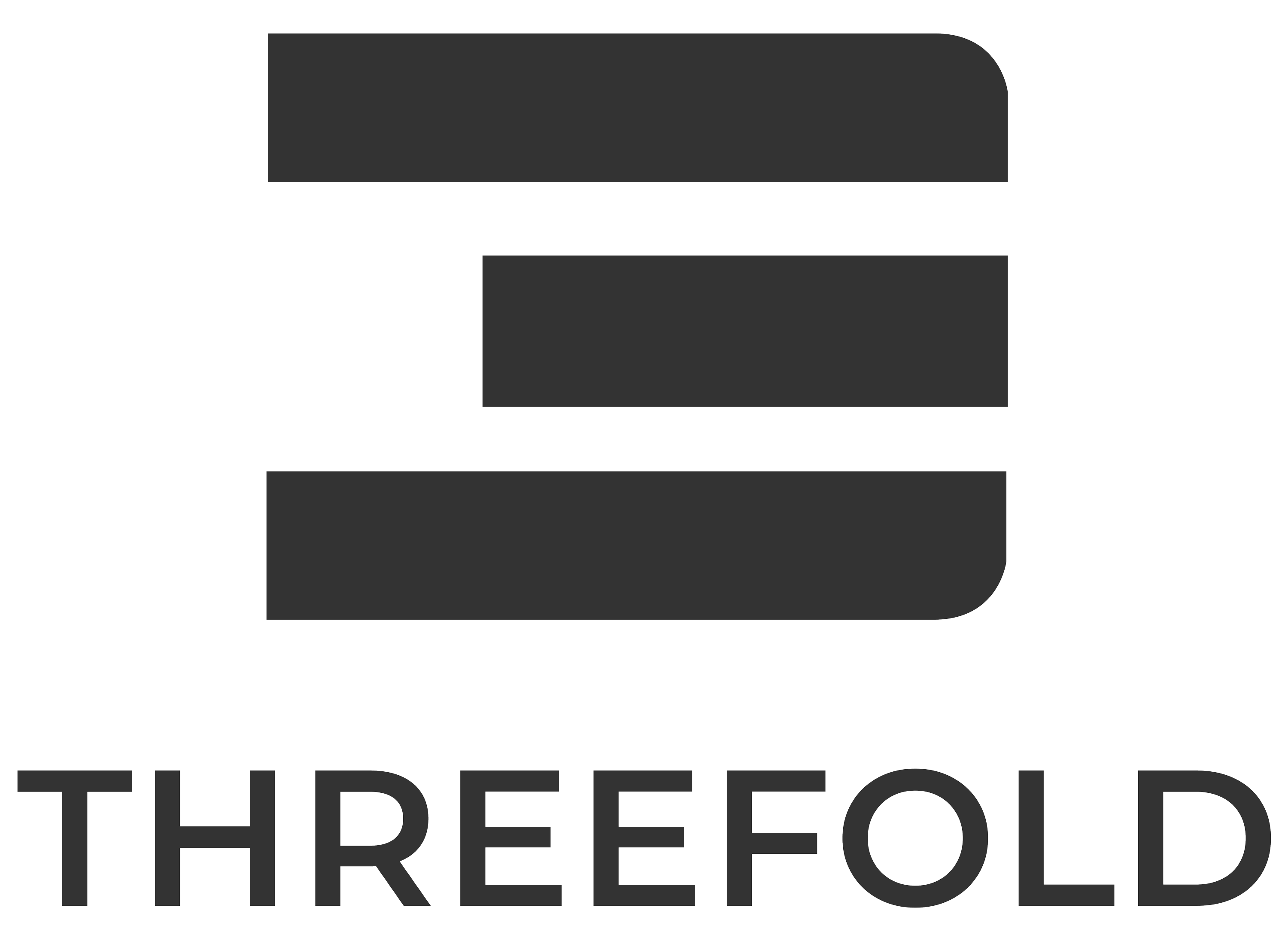
Threefold Grid v0.8.1 published on Wednesday, Nov 6, 2024 by Threefold
Create Network Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Network(name: string, args: NetworkArgs, opts?: CustomResourceOptions);
@overload
def Network(resource_name: str,
args: NetworkArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Network(resource_name: str,
opts: Optional[ResourceOptions] = None,
description: Optional[str] = None,
ip_range: Optional[str] = None,
name: Optional[str] = None,
nodes: Optional[Sequence[Any]] = None,
add_wg_access: Optional[bool] = None,
mycelium: Optional[bool] = None,
mycelium_keys: Optional[Mapping[str, str]] = None,
solution_type: Optional[str] = None)
func NewNetwork(ctx *Context, name string, args NetworkArgs, opts ...ResourceOption) (*Network, error)
public Network(string name, NetworkArgs args, CustomResourceOptions? opts = null)
public Network(String name, NetworkArgs args)
public Network(String name, NetworkArgs args, CustomResourceOptions options)
type: threefold:Network
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args NetworkArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args NetworkArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args NetworkArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args NetworkArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args NetworkArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var networkResource = new Threefold.Network("networkResource", new()
{
Description = "string",
Ip_range = "string",
Name = "string",
Nodes = new[]
{
"any",
},
Add_wg_access = false,
Mycelium = false,
Mycelium_keys =
{
{ "string", "string" },
},
Solution_type = "string",
});
example, err := threefold.NewNetwork(ctx, "networkResource", &threefold.NetworkArgs{
Description: pulumi.String("string"),
Ip_range: pulumi.String("string"),
Name: pulumi.String("string"),
Nodes: pulumi.Array{
pulumi.Any("any"),
},
Add_wg_access: pulumi.Bool(false),
Mycelium: pulumi.Bool(false),
Mycelium_keys: pulumi.StringMap{
"string": pulumi.String("string"),
},
Solution_type: pulumi.String("string"),
})
var networkResource = new Network("networkResource", NetworkArgs.builder()
.description("string")
.ip_range("string")
.name("string")
.nodes("any")
.add_wg_access(false)
.mycelium(false)
.mycelium_keys(Map.of("string", "string"))
.solution_type("string")
.build());
network_resource = threefold.Network("networkResource",
description="string",
ip_range="string",
name="string",
nodes=["any"],
add_wg_access=False,
mycelium=False,
mycelium_keys={
"string": "string",
},
solution_type="string")
const networkResource = new threefold.Network("networkResource", {
description: "string",
ip_range: "string",
name: "string",
nodes: ["any"],
add_wg_access: false,
mycelium: false,
mycelium_keys: {
string: "string",
},
solution_type: "string",
});
type: threefold:Network
properties:
add_wg_access: false
description: string
ip_range: string
mycelium: false
mycelium_keys:
string: string
name: string
nodes:
- any
solution_type: string
Network Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
In Python, inputs that are objects can be passed either as argument classes or as dictionary literals.
The Network resource accepts the following input properties:
- Description string
- The description of the network workload, optional with no restrictions
- Ip_
range string - The IP range for the network, subnet should be 16
- Name string
- The name of the network workload, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- Nodes List<object>
- The nodes used to deploy the network on, shouldn't be empty
- Add_
wg_ boolaccess - A flag to support wireguard in the network
- Mycelium bool
- A flag to generate a random mycelium key to support mycelium in the network
- Mycelium_
keys Dictionary<string, string> - A map of nodes as a key and mycelium key for each node, mycelium key length should be 32. Selected nodes must be included in the network's nodes
- Solution_
type string - The solution type of the network, displayed as project name in contract metadata
- Description string
- The description of the network workload, optional with no restrictions
- Ip_
range string - The IP range for the network, subnet should be 16
- Name string
- The name of the network workload, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- Nodes []interface{}
- The nodes used to deploy the network on, shouldn't be empty
- Add_
wg_ boolaccess - A flag to support wireguard in the network
- Mycelium bool
- A flag to generate a random mycelium key to support mycelium in the network
- Mycelium_
keys map[string]string - A map of nodes as a key and mycelium key for each node, mycelium key length should be 32. Selected nodes must be included in the network's nodes
- Solution_
type string - The solution type of the network, displayed as project name in contract metadata
- description String
- The description of the network workload, optional with no restrictions
- ip_
range String - The IP range for the network, subnet should be 16
- name String
- The name of the network workload, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- nodes List<Object>
- The nodes used to deploy the network on, shouldn't be empty
- add_
wg_ Booleanaccess - A flag to support wireguard in the network
- mycelium Boolean
- A flag to generate a random mycelium key to support mycelium in the network
- mycelium_
keys Map<String,String> - A map of nodes as a key and mycelium key for each node, mycelium key length should be 32. Selected nodes must be included in the network's nodes
- solution_
type String - The solution type of the network, displayed as project name in contract metadata
- description string
- The description of the network workload, optional with no restrictions
- ip_
range string - The IP range for the network, subnet should be 16
- name string
- The name of the network workload, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- nodes any[]
- The nodes used to deploy the network on, shouldn't be empty
- add_
wg_ booleanaccess - A flag to support wireguard in the network
- mycelium boolean
- A flag to generate a random mycelium key to support mycelium in the network
- mycelium_
keys {[key: string]: string} - A map of nodes as a key and mycelium key for each node, mycelium key length should be 32. Selected nodes must be included in the network's nodes
- solution_
type string - The solution type of the network, displayed as project name in contract metadata
- description str
- The description of the network workload, optional with no restrictions
- ip_
range str - The IP range for the network, subnet should be 16
- name str
- The name of the network workload, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- nodes Sequence[Any]
- The nodes used to deploy the network on, shouldn't be empty
- add_
wg_ boolaccess - A flag to support wireguard in the network
- mycelium bool
- A flag to generate a random mycelium key to support mycelium in the network
- mycelium_
keys Mapping[str, str] - A map of nodes as a key and mycelium key for each node, mycelium key length should be 32. Selected nodes must be included in the network's nodes
- solution_
type str - The solution type of the network, displayed as project name in contract metadata
- description String
- The description of the network workload, optional with no restrictions
- ip_
range String - The IP range for the network, subnet should be 16
- name String
- The name of the network workload, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- nodes List<Any>
- The nodes used to deploy the network on, shouldn't be empty
- add_
wg_ Booleanaccess - A flag to support wireguard in the network
- mycelium Boolean
- A flag to generate a random mycelium key to support mycelium in the network
- mycelium_
keys Map<String> - A map of nodes as a key and mycelium key for each node, mycelium key length should be 32. Selected nodes must be included in the network's nodes
- solution_
type String - The solution type of the network, displayed as project name in contract metadata
Outputs
All input properties are implicitly available as output properties. Additionally, the Network resource produces the following output properties:
- Access_
wg_ stringconfig - Generated wireguard configuration for external user access to the network
- External_
ip string - Wireguard IP assigned for external user access
- External_
sk string - External user private key used in encryption while communicating through Wireguard network
- Id string
- The provider-assigned unique ID for this managed resource.
- Node_
deployment_ Dictionary<string, int>id - Mapping from each node to its deployment id
- Nodes_
ip_ Dictionary<string, string>range - Computed values of nodes' IP ranges after deployment
- Public_
node_ intid - Public node id (in case it's added). Used for wireguard access and supporting hidden nodes
- Access_
wg_ stringconfig - Generated wireguard configuration for external user access to the network
- External_
ip string - Wireguard IP assigned for external user access
- External_
sk string - External user private key used in encryption while communicating through Wireguard network
- Id string
- The provider-assigned unique ID for this managed resource.
- Node_
deployment_ map[string]intid - Mapping from each node to its deployment id
- Nodes_
ip_ map[string]stringrange - Computed values of nodes' IP ranges after deployment
- Public_
node_ intid - Public node id (in case it's added). Used for wireguard access and supporting hidden nodes
- access_
wg_ Stringconfig - Generated wireguard configuration for external user access to the network
- external_
ip String - Wireguard IP assigned for external user access
- external_
sk String - External user private key used in encryption while communicating through Wireguard network
- id String
- The provider-assigned unique ID for this managed resource.
- node_
deployment_ Map<String,Integer>id - Mapping from each node to its deployment id
- nodes_
ip_ Map<String,String>range - Computed values of nodes' IP ranges after deployment
- public_
node_ Integerid - Public node id (in case it's added). Used for wireguard access and supporting hidden nodes
- access_
wg_ stringconfig - Generated wireguard configuration for external user access to the network
- external_
ip string - Wireguard IP assigned for external user access
- external_
sk string - External user private key used in encryption while communicating through Wireguard network
- id string
- The provider-assigned unique ID for this managed resource.
- node_
deployment_ {[key: string]: number}id - Mapping from each node to its deployment id
- nodes_
ip_ {[key: string]: string}range - Computed values of nodes' IP ranges after deployment
- public_
node_ numberid - Public node id (in case it's added). Used for wireguard access and supporting hidden nodes
- access_
wg_ strconfig - Generated wireguard configuration for external user access to the network
- external_
ip str - Wireguard IP assigned for external user access
- external_
sk str - External user private key used in encryption while communicating through Wireguard network
- id str
- The provider-assigned unique ID for this managed resource.
- node_
deployment_ Mapping[str, int]id - Mapping from each node to its deployment id
- nodes_
ip_ Mapping[str, str]range - Computed values of nodes' IP ranges after deployment
- public_
node_ intid - Public node id (in case it's added). Used for wireguard access and supporting hidden nodes
- access_
wg_ Stringconfig - Generated wireguard configuration for external user access to the network
- external_
ip String - Wireguard IP assigned for external user access
- external_
sk String - External user private key used in encryption while communicating through Wireguard network
- id String
- The provider-assigned unique ID for this managed resource.
- node_
deployment_ Map<Number>id - Mapping from each node to its deployment id
- nodes_
ip_ Map<String>range - Computed values of nodes' IP ranges after deployment
- public_
node_ Numberid - Public node id (in case it's added). Used for wireguard access and supporting hidden nodes
Package Details
- Repository
- threefold threefoldtech/pulumi-threefold
- License
- Apache-2.0
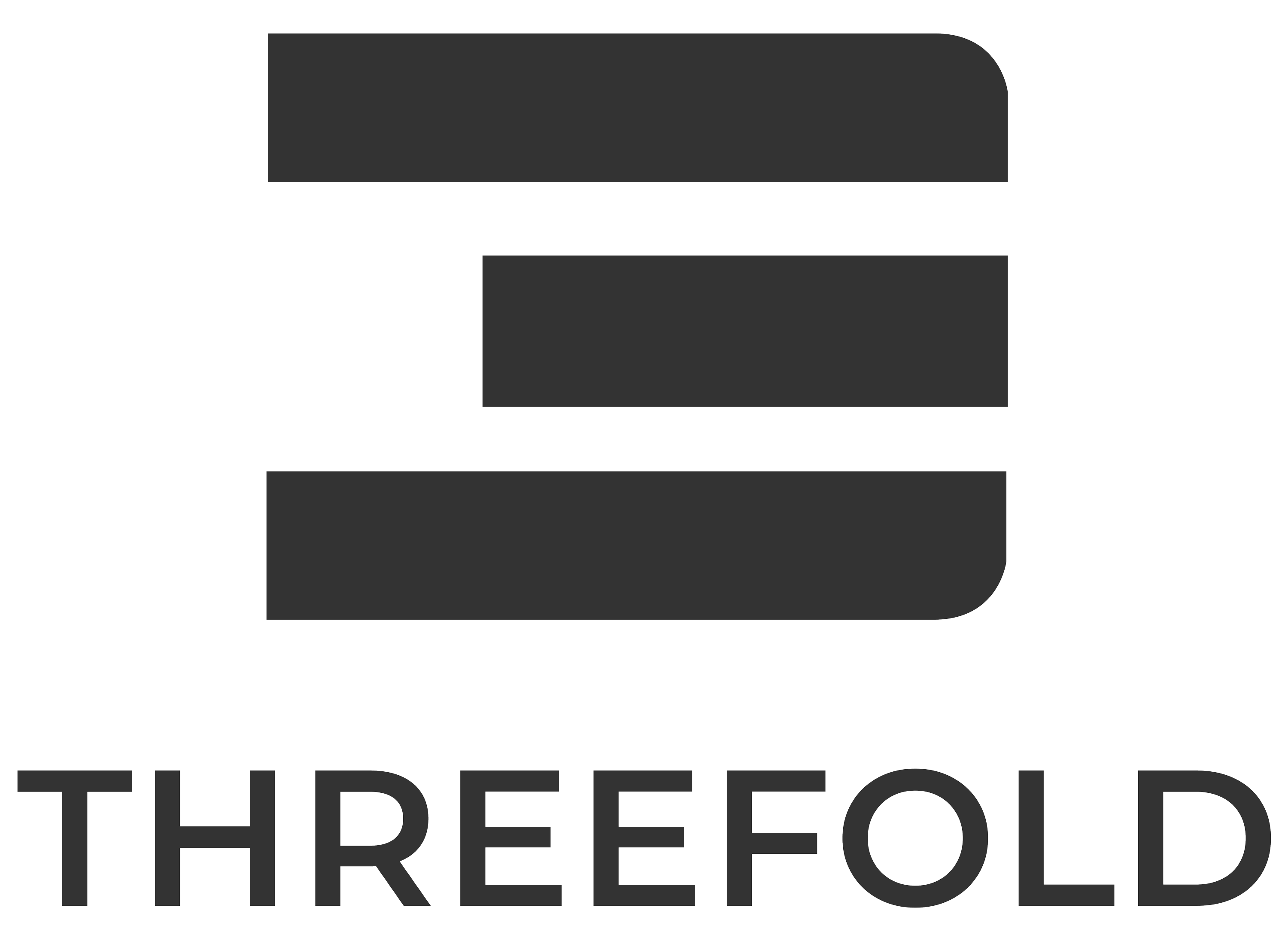
Threefold Grid v0.8.1 published on Wednesday, Nov 6, 2024 by Threefold