Threefold Grid v0.8.1 published on Wednesday, Nov 6, 2024 by Threefold
threefold.Kubernetes
Explore with Pulumi AI
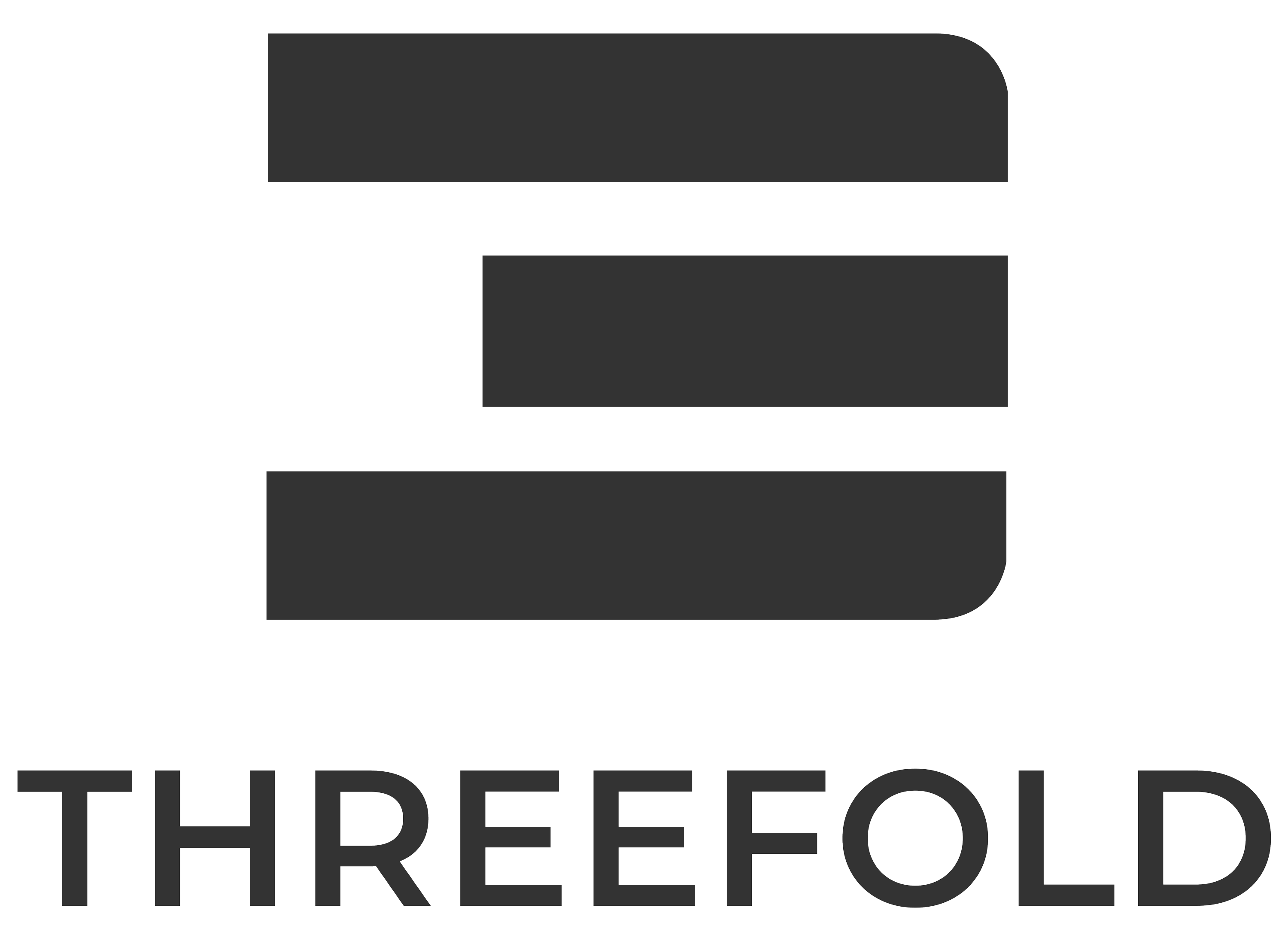
Threefold Grid v0.8.1 published on Wednesday, Nov 6, 2024 by Threefold
Create Kubernetes Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Kubernetes(name: string, args: KubernetesArgs, opts?: CustomResourceOptions);
@overload
def Kubernetes(resource_name: str,
args: KubernetesArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Kubernetes(resource_name: str,
opts: Optional[ResourceOptions] = None,
master: Optional[K8sNodeInputArgs] = None,
network_name: Optional[str] = None,
token: Optional[str] = None,
workers: Optional[Sequence[K8sNodeInputArgs]] = None,
entry_point: Optional[str] = None,
flist: Optional[str] = None,
flist_checksum: Optional[str] = None,
solution_type: Optional[str] = None,
ssh_key: Optional[str] = None)
func NewKubernetes(ctx *Context, name string, args KubernetesArgs, opts ...ResourceOption) (*Kubernetes, error)
public Kubernetes(string name, KubernetesArgs args, CustomResourceOptions? opts = null)
public Kubernetes(String name, KubernetesArgs args)
public Kubernetes(String name, KubernetesArgs args, CustomResourceOptions options)
type: threefold:Kubernetes
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var kubernetesResource = new Threefold.Kubernetes("kubernetesResource", new()
{
Master = new Threefold.Inputs.K8sNodeInputArgs
{
Name = "string",
Memory = 0,
Disk_size = 0,
Node_id = "any",
Network_name = "string",
Cpu = 0,
Flist = "string",
Mycelium_ip_seed = "string",
Description = "string",
Flist_checksum = "string",
Mycelium = false,
Entry_point = "string",
Planetary = false,
Public_ip = false,
Public_ip6 = false,
},
Network_name = "string",
Token = "string",
Workers = new[]
{
new Threefold.Inputs.K8sNodeInputArgs
{
Name = "string",
Memory = 0,
Disk_size = 0,
Node_id = "any",
Network_name = "string",
Cpu = 0,
Flist = "string",
Mycelium_ip_seed = "string",
Description = "string",
Flist_checksum = "string",
Mycelium = false,
Entry_point = "string",
Planetary = false,
Public_ip = false,
Public_ip6 = false,
},
},
Entry_point = "string",
Flist = "string",
Flist_checksum = "string",
Solution_type = "string",
Ssh_key = "string",
});
example, err := threefold.NewKubernetes(ctx, "kubernetesResource", &threefold.KubernetesArgs{
Master: &threefold.K8sNodeInputArgs{
Name: pulumi.String("string"),
Memory: pulumi.Int(0),
Disk_size: pulumi.Int(0),
Node_id: pulumi.Any("any"),
Network_name: pulumi.String("string"),
Cpu: pulumi.Int(0),
Flist: pulumi.String("string"),
Mycelium_ip_seed: pulumi.String("string"),
Description: pulumi.String("string"),
Flist_checksum: pulumi.String("string"),
Mycelium: pulumi.Bool(false),
Entry_point: pulumi.String("string"),
Planetary: pulumi.Bool(false),
Public_ip: pulumi.Bool(false),
Public_ip6: pulumi.Bool(false),
},
Network_name: pulumi.String("string"),
Token: pulumi.String("string"),
Workers: threefold.K8sNodeInputArray{
&threefold.K8sNodeInputArgs{
Name: pulumi.String("string"),
Memory: pulumi.Int(0),
Disk_size: pulumi.Int(0),
Node_id: pulumi.Any("any"),
Network_name: pulumi.String("string"),
Cpu: pulumi.Int(0),
Flist: pulumi.String("string"),
Mycelium_ip_seed: pulumi.String("string"),
Description: pulumi.String("string"),
Flist_checksum: pulumi.String("string"),
Mycelium: pulumi.Bool(false),
Entry_point: pulumi.String("string"),
Planetary: pulumi.Bool(false),
Public_ip: pulumi.Bool(false),
Public_ip6: pulumi.Bool(false),
},
},
Entry_point: pulumi.String("string"),
Flist: pulumi.String("string"),
Flist_checksum: pulumi.String("string"),
Solution_type: pulumi.String("string"),
Ssh_key: pulumi.String("string"),
})
var kubernetesResource = new Kubernetes("kubernetesResource", KubernetesArgs.builder()
.master(K8sNodeInputArgs.builder()
.name("string")
.memory(0)
.disk_size(0)
.node_id("any")
.network_name("string")
.cpu(0)
.flist("string")
.mycelium_ip_seed("string")
.description("string")
.flist_checksum("string")
.mycelium(false)
.entry_point("string")
.planetary(false)
.public_ip(false)
.public_ip6(false)
.build())
.network_name("string")
.token("string")
.workers(K8sNodeInputArgs.builder()
.name("string")
.memory(0)
.disk_size(0)
.node_id("any")
.network_name("string")
.cpu(0)
.flist("string")
.mycelium_ip_seed("string")
.description("string")
.flist_checksum("string")
.mycelium(false)
.entry_point("string")
.planetary(false)
.public_ip(false)
.public_ip6(false)
.build())
.entry_point("string")
.flist("string")
.flist_checksum("string")
.solution_type("string")
.ssh_key("string")
.build());
kubernetes_resource = threefold.Kubernetes("kubernetesResource",
master={
"name": "string",
"memory": 0,
"disk_size": 0,
"node_id": "any",
"network_name": "string",
"cpu": 0,
"flist": "string",
"mycelium_ip_seed": "string",
"description": "string",
"flist_checksum": "string",
"mycelium": False,
"entry_point": "string",
"planetary": False,
"public_ip": False,
"public_ip6": False,
},
network_name="string",
token="string",
workers=[{
"name": "string",
"memory": 0,
"disk_size": 0,
"node_id": "any",
"network_name": "string",
"cpu": 0,
"flist": "string",
"mycelium_ip_seed": "string",
"description": "string",
"flist_checksum": "string",
"mycelium": False,
"entry_point": "string",
"planetary": False,
"public_ip": False,
"public_ip6": False,
}],
entry_point="string",
flist="string",
flist_checksum="string",
solution_type="string",
ssh_key="string")
const kubernetesResource = new threefold.Kubernetes("kubernetesResource", {
master: {
name: "string",
memory: 0,
disk_size: 0,
node_id: "any",
network_name: "string",
cpu: 0,
flist: "string",
mycelium_ip_seed: "string",
description: "string",
flist_checksum: "string",
mycelium: false,
entry_point: "string",
planetary: false,
public_ip: false,
public_ip6: false,
},
network_name: "string",
token: "string",
workers: [{
name: "string",
memory: 0,
disk_size: 0,
node_id: "any",
network_name: "string",
cpu: 0,
flist: "string",
mycelium_ip_seed: "string",
description: "string",
flist_checksum: "string",
mycelium: false,
entry_point: "string",
planetary: false,
public_ip: false,
public_ip6: false,
}],
entry_point: "string",
flist: "string",
flist_checksum: "string",
solution_type: "string",
ssh_key: "string",
});
type: threefold:Kubernetes
properties:
entry_point: string
flist: string
flist_checksum: string
master:
cpu: 0
description: string
disk_size: 0
entry_point: string
flist: string
flist_checksum: string
memory: 0
mycelium: false
mycelium_ip_seed: string
name: string
network_name: string
node_id: any
planetary: false
public_ip: false
public_ip6: false
network_name: string
solution_type: string
ssh_key: string
token: string
workers:
- cpu: 0
description: string
disk_size: 0
entry_point: string
flist: string
flist_checksum: string
memory: 0
mycelium: false
mycelium_ip_seed: string
name: string
network_name: string
node_id: any
planetary: false
public_ip: false
public_ip6: false
Kubernetes Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
In Python, inputs that are objects can be passed either as argument classes or as dictionary literals.
The Kubernetes resource accepts the following input properties:
- Master
K8s
Node Input - Master holds the configuration of master node in the kubernetes cluster
- Network_
name string - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- Token string
- The cluster secret token. Each node has to have this token to be part of the cluster. This token should be an alphanumeric non-empty string
- Workers
List<K8s
Node Input> - Workers is a list holding the workers configuration for the kubernetes cluster
- Entry_
point string - The entry point for the flist. Example: /sbin/zinit init
- Flist string
- The flist to be mounted in the kubernetes cluster nodes. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- Flist_
checksum string - The checksum of the flist which should match the checksum of the given flist, optional
- Solution_
type string - The solution type of the cluster, displayed as project name in contract metadata
- Ssh_
key string - SSH key to access the cluster nodes
- Master
K8s
Node Input Args - Master holds the configuration of master node in the kubernetes cluster
- Network_
name string - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- Token string
- The cluster secret token. Each node has to have this token to be part of the cluster. This token should be an alphanumeric non-empty string
- Workers
[]K8s
Node Input Args - Workers is a list holding the workers configuration for the kubernetes cluster
- Entry_
point string - The entry point for the flist. Example: /sbin/zinit init
- Flist string
- The flist to be mounted in the kubernetes cluster nodes. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- Flist_
checksum string - The checksum of the flist which should match the checksum of the given flist, optional
- Solution_
type string - The solution type of the cluster, displayed as project name in contract metadata
- Ssh_
key string - SSH key to access the cluster nodes
- master
K8s
Node Input - Master holds the configuration of master node in the kubernetes cluster
- network_
name String - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- token String
- The cluster secret token. Each node has to have this token to be part of the cluster. This token should be an alphanumeric non-empty string
- workers
List<K8s
Node Input> - Workers is a list holding the workers configuration for the kubernetes cluster
- entry_
point String - The entry point for the flist. Example: /sbin/zinit init
- flist String
- The flist to be mounted in the kubernetes cluster nodes. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- flist_
checksum String - The checksum of the flist which should match the checksum of the given flist, optional
- solution_
type String - The solution type of the cluster, displayed as project name in contract metadata
- ssh_
key String - SSH key to access the cluster nodes
- master
K8s
Node Input - Master holds the configuration of master node in the kubernetes cluster
- network_
name string - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- token string
- The cluster secret token. Each node has to have this token to be part of the cluster. This token should be an alphanumeric non-empty string
- workers
K8s
Node Input[] - Workers is a list holding the workers configuration for the kubernetes cluster
- entry_
point string - The entry point for the flist. Example: /sbin/zinit init
- flist string
- The flist to be mounted in the kubernetes cluster nodes. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- flist_
checksum string - The checksum of the flist which should match the checksum of the given flist, optional
- solution_
type string - The solution type of the cluster, displayed as project name in contract metadata
- ssh_
key string - SSH key to access the cluster nodes
- master
K8s
Node Input Args - Master holds the configuration of master node in the kubernetes cluster
- network_
name str - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- token str
- The cluster secret token. Each node has to have this token to be part of the cluster. This token should be an alphanumeric non-empty string
- workers
Sequence[K8s
Node Input Args] - Workers is a list holding the workers configuration for the kubernetes cluster
- entry_
point str - The entry point for the flist. Example: /sbin/zinit init
- flist str
- The flist to be mounted in the kubernetes cluster nodes. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- flist_
checksum str - The checksum of the flist which should match the checksum of the given flist, optional
- solution_
type str - The solution type of the cluster, displayed as project name in contract metadata
- ssh_
key str - SSH key to access the cluster nodes
- master Property Map
- Master holds the configuration of master node in the kubernetes cluster
- network_
name String - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- token String
- The cluster secret token. Each node has to have this token to be part of the cluster. This token should be an alphanumeric non-empty string
- workers List<Property Map>
- Workers is a list holding the workers configuration for the kubernetes cluster
- entry_
point String - The entry point for the flist. Example: /sbin/zinit init
- flist String
- The flist to be mounted in the kubernetes cluster nodes. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- flist_
checksum String - The checksum of the flist which should match the checksum of the given flist, optional
- solution_
type String - The solution type of the cluster, displayed as project name in contract metadata
- ssh_
key String - SSH key to access the cluster nodes
Outputs
All input properties are implicitly available as output properties. Additionally, the Kubernetes resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Master_
computed VMComputed - The computed fields of the master node
- Node_
deployment_ Dictionary<string, int>id - Mapping from each node to its deployment ID
- Nodes_
ip_ Dictionary<string, string>range - Computed values of nodes' IP ranges after deployment
- Workers_
computed Dictionary<string, VMComputed> - List of the computed fields of the worker nodes
- Id string
- The provider-assigned unique ID for this managed resource.
- Master_
computed VMComputed - The computed fields of the master node
- Node_
deployment_ map[string]intid - Mapping from each node to its deployment ID
- Nodes_
ip_ map[string]stringrange - Computed values of nodes' IP ranges after deployment
- Workers_
computed map[string]VMComputed - List of the computed fields of the worker nodes
- id String
- The provider-assigned unique ID for this managed resource.
- master_
computed VMComputed - The computed fields of the master node
- node_
deployment_ Map<String,Integer>id - Mapping from each node to its deployment ID
- nodes_
ip_ Map<String,String>range - Computed values of nodes' IP ranges after deployment
- workers_
computed Map<String,VMComputed> - List of the computed fields of the worker nodes
- id string
- The provider-assigned unique ID for this managed resource.
- master_
computed VMComputed - The computed fields of the master node
- node_
deployment_ {[key: string]: number}id - Mapping from each node to its deployment ID
- nodes_
ip_ {[key: string]: string}range - Computed values of nodes' IP ranges after deployment
- workers_
computed {[key: string]: VMComputed} - List of the computed fields of the worker nodes
- id str
- The provider-assigned unique ID for this managed resource.
- master_
computed VMComputed - The computed fields of the master node
- node_
deployment_ Mapping[str, int]id - Mapping from each node to its deployment ID
- nodes_
ip_ Mapping[str, str]range - Computed values of nodes' IP ranges after deployment
- workers_
computed Mapping[str, VMComputed] - List of the computed fields of the worker nodes
- id String
- The provider-assigned unique ID for this managed resource.
- master_
computed Property Map - The computed fields of the master node
- node_
deployment_ Map<Number>id - Mapping from each node to its deployment ID
- nodes_
ip_ Map<String>range - Computed values of nodes' IP ranges after deployment
- workers_
computed Map<Property Map> - List of the computed fields of the worker nodes
Supporting Types
K8sNodeInput, K8sNodeInputArgs
- Cpu int
- The cpu units needed for the kubernetes node. Range in [1: 32]
- Disk_
size int - Data disk size in GBs. Must be between 1GB and 10240GBs (10TBs)
- Memory int
- The memory capacity for the kubernetes node in MB. Min is 250 MB
- Name string
- The name of the kubernetes node, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- Network_
name string - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- Node_
id object - The node ID to deploy the kubernetes node on, required and should match the requested resources
- Description string
- The description of the kubernetes node, optional with no restrictions
- Entry_
point string - The entry point for the flist. Example: /sbin/zinit init
- Flist string
- The flist to be mounted in the kubernetes node. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- Flist_
checksum string - The checksum of the flist which should match the checksum of the given flist, optional
- Mycelium bool
- A flag to generate a random mycelium IP seed to support mycelium in the kubernetes node
- Mycelium_
ip_ stringseed - The seed used for mycelium IP generated for the kubernetes node. It's length should be 6
- Planetary bool
- A flag to enable generating a yggdrasil IP for the kubernetes node
- Public_
ip bool - A flag to enable generating a public IP for the kubernetes node, public node is required for it
- Public_
ip6 bool - A flag to enable generating a public IPv6 for the kubernetes node, public node is required for it
- Cpu int
- The cpu units needed for the kubernetes node. Range in [1: 32]
- Disk_
size int - Data disk size in GBs. Must be between 1GB and 10240GBs (10TBs)
- Memory int
- The memory capacity for the kubernetes node in MB. Min is 250 MB
- Name string
- The name of the kubernetes node, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- Network_
name string - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- Node_
id interface{} - The node ID to deploy the kubernetes node on, required and should match the requested resources
- Description string
- The description of the kubernetes node, optional with no restrictions
- Entry_
point string - The entry point for the flist. Example: /sbin/zinit init
- Flist string
- The flist to be mounted in the kubernetes node. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- Flist_
checksum string - The checksum of the flist which should match the checksum of the given flist, optional
- Mycelium bool
- A flag to generate a random mycelium IP seed to support mycelium in the kubernetes node
- Mycelium_
ip_ stringseed - The seed used for mycelium IP generated for the kubernetes node. It's length should be 6
- Planetary bool
- A flag to enable generating a yggdrasil IP for the kubernetes node
- Public_
ip bool - A flag to enable generating a public IP for the kubernetes node, public node is required for it
- Public_
ip6 bool - A flag to enable generating a public IPv6 for the kubernetes node, public node is required for it
- cpu Integer
- The cpu units needed for the kubernetes node. Range in [1: 32]
- disk_
size Integer - Data disk size in GBs. Must be between 1GB and 10240GBs (10TBs)
- memory Integer
- The memory capacity for the kubernetes node in MB. Min is 250 MB
- name String
- The name of the kubernetes node, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- network_
name String - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- node_
id Object - The node ID to deploy the kubernetes node on, required and should match the requested resources
- description String
- The description of the kubernetes node, optional with no restrictions
- entry_
point String - The entry point for the flist. Example: /sbin/zinit init
- flist String
- The flist to be mounted in the kubernetes node. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- flist_
checksum String - The checksum of the flist which should match the checksum of the given flist, optional
- mycelium Boolean
- A flag to generate a random mycelium IP seed to support mycelium in the kubernetes node
- mycelium_
ip_ Stringseed - The seed used for mycelium IP generated for the kubernetes node. It's length should be 6
- planetary Boolean
- A flag to enable generating a yggdrasil IP for the kubernetes node
- public_
ip Boolean - A flag to enable generating a public IP for the kubernetes node, public node is required for it
- public_
ip6 Boolean - A flag to enable generating a public IPv6 for the kubernetes node, public node is required for it
- cpu number
- The cpu units needed for the kubernetes node. Range in [1: 32]
- disk_
size number - Data disk size in GBs. Must be between 1GB and 10240GBs (10TBs)
- memory number
- The memory capacity for the kubernetes node in MB. Min is 250 MB
- name string
- The name of the kubernetes node, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- network_
name string - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- node_
id any - The node ID to deploy the kubernetes node on, required and should match the requested resources
- description string
- The description of the kubernetes node, optional with no restrictions
- entry_
point string - The entry point for the flist. Example: /sbin/zinit init
- flist string
- The flist to be mounted in the kubernetes node. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- flist_
checksum string - The checksum of the flist which should match the checksum of the given flist, optional
- mycelium boolean
- A flag to generate a random mycelium IP seed to support mycelium in the kubernetes node
- mycelium_
ip_ stringseed - The seed used for mycelium IP generated for the kubernetes node. It's length should be 6
- planetary boolean
- A flag to enable generating a yggdrasil IP for the kubernetes node
- public_
ip boolean - A flag to enable generating a public IP for the kubernetes node, public node is required for it
- public_
ip6 boolean - A flag to enable generating a public IPv6 for the kubernetes node, public node is required for it
- cpu int
- The cpu units needed for the kubernetes node. Range in [1: 32]
- disk_
size int - Data disk size in GBs. Must be between 1GB and 10240GBs (10TBs)
- memory int
- The memory capacity for the kubernetes node in MB. Min is 250 MB
- name str
- The name of the kubernetes node, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- network_
name str - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- node_
id Any - The node ID to deploy the kubernetes node on, required and should match the requested resources
- description str
- The description of the kubernetes node, optional with no restrictions
- entry_
point str - The entry point for the flist. Example: /sbin/zinit init
- flist str
- The flist to be mounted in the kubernetes node. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- flist_
checksum str - The checksum of the flist which should match the checksum of the given flist, optional
- mycelium bool
- A flag to generate a random mycelium IP seed to support mycelium in the kubernetes node
- mycelium_
ip_ strseed - The seed used for mycelium IP generated for the kubernetes node. It's length should be 6
- planetary bool
- A flag to enable generating a yggdrasil IP for the kubernetes node
- public_
ip bool - A flag to enable generating a public IP for the kubernetes node, public node is required for it
- public_
ip6 bool - A flag to enable generating a public IPv6 for the kubernetes node, public node is required for it
- cpu Number
- The cpu units needed for the kubernetes node. Range in [1: 32]
- disk_
size Number - Data disk size in GBs. Must be between 1GB and 10240GBs (10TBs)
- memory Number
- The memory capacity for the kubernetes node in MB. Min is 250 MB
- name String
- The name of the kubernetes node, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported
- network_
name String - The name of the network, it's required and cannot exceed 50 characters. Only alphanumeric and underscores characters are supported. Network must exist
- node_
id Any - The node ID to deploy the kubernetes node on, required and should match the requested resources
- description String
- The description of the kubernetes node, optional with no restrictions
- entry_
point String - The entry point for the flist. Example: /sbin/zinit init
- flist String
- The flist to be mounted in the kubernetes node. Example: https://hub.grid.tf/tf-official-apps/base:latest.flist
- flist_
checksum String - The checksum of the flist which should match the checksum of the given flist, optional
- mycelium Boolean
- A flag to generate a random mycelium IP seed to support mycelium in the kubernetes node
- mycelium_
ip_ Stringseed - The seed used for mycelium IP generated for the kubernetes node. It's length should be 6
- planetary Boolean
- A flag to enable generating a yggdrasil IP for the kubernetes node
- public_
ip Boolean - A flag to enable generating a public IP for the kubernetes node, public node is required for it
- public_
ip6 Boolean - A flag to enable generating a public IPv6 for the kubernetes node, public node is required for it
VMComputed, VMComputedArgs
- Computed_
ip string - The reserved public ipv4 if any
- Computed_
ip6 string - The reserved public ipv6 if any
- Console_
url string - The url to access the vm via cloud console on private interface using wireguard
- Mycelium_
ip string - The allocated mycelium IP
- Mycelium_
ip_ stringseed - The seed used for mycelium IP generated for the virtual machine. It's length should be 6
- Planetary_
ip string - The allocated Yggdrasil IP
- Ip string
- The private wireguard IP of the vm
- Computed_
ip string - The reserved public ipv4 if any
- Computed_
ip6 string - The reserved public ipv6 if any
- Console_
url string - The url to access the vm via cloud console on private interface using wireguard
- Mycelium_
ip string - The allocated mycelium IP
- Mycelium_
ip_ stringseed - The seed used for mycelium IP generated for the virtual machine. It's length should be 6
- Planetary_
ip string - The allocated Yggdrasil IP
- Ip string
- The private wireguard IP of the vm
- computed_
ip String - The reserved public ipv4 if any
- computed_
ip6 String - The reserved public ipv6 if any
- console_
url String - The url to access the vm via cloud console on private interface using wireguard
- mycelium_
ip String - The allocated mycelium IP
- mycelium_
ip_ Stringseed - The seed used for mycelium IP generated for the virtual machine. It's length should be 6
- planetary_
ip String - The allocated Yggdrasil IP
- ip String
- The private wireguard IP of the vm
- computed_
ip string - The reserved public ipv4 if any
- computed_
ip6 string - The reserved public ipv6 if any
- console_
url string - The url to access the vm via cloud console on private interface using wireguard
- mycelium_
ip string - The allocated mycelium IP
- mycelium_
ip_ stringseed - The seed used for mycelium IP generated for the virtual machine. It's length should be 6
- planetary_
ip string - The allocated Yggdrasil IP
- ip string
- The private wireguard IP of the vm
- computed_
ip str - The reserved public ipv4 if any
- computed_
ip6 str - The reserved public ipv6 if any
- console_
url str - The url to access the vm via cloud console on private interface using wireguard
- mycelium_
ip str - The allocated mycelium IP
- mycelium_
ip_ strseed - The seed used for mycelium IP generated for the virtual machine. It's length should be 6
- planetary_
ip str - The allocated Yggdrasil IP
- ip str
- The private wireguard IP of the vm
- computed_
ip String - The reserved public ipv4 if any
- computed_
ip6 String - The reserved public ipv6 if any
- console_
url String - The url to access the vm via cloud console on private interface using wireguard
- mycelium_
ip String - The allocated mycelium IP
- mycelium_
ip_ Stringseed - The seed used for mycelium IP generated for the virtual machine. It's length should be 6
- planetary_
ip String - The allocated Yggdrasil IP
- ip String
- The private wireguard IP of the vm
Package Details
- Repository
- threefold threefoldtech/pulumi-threefold
- License
- Apache-2.0
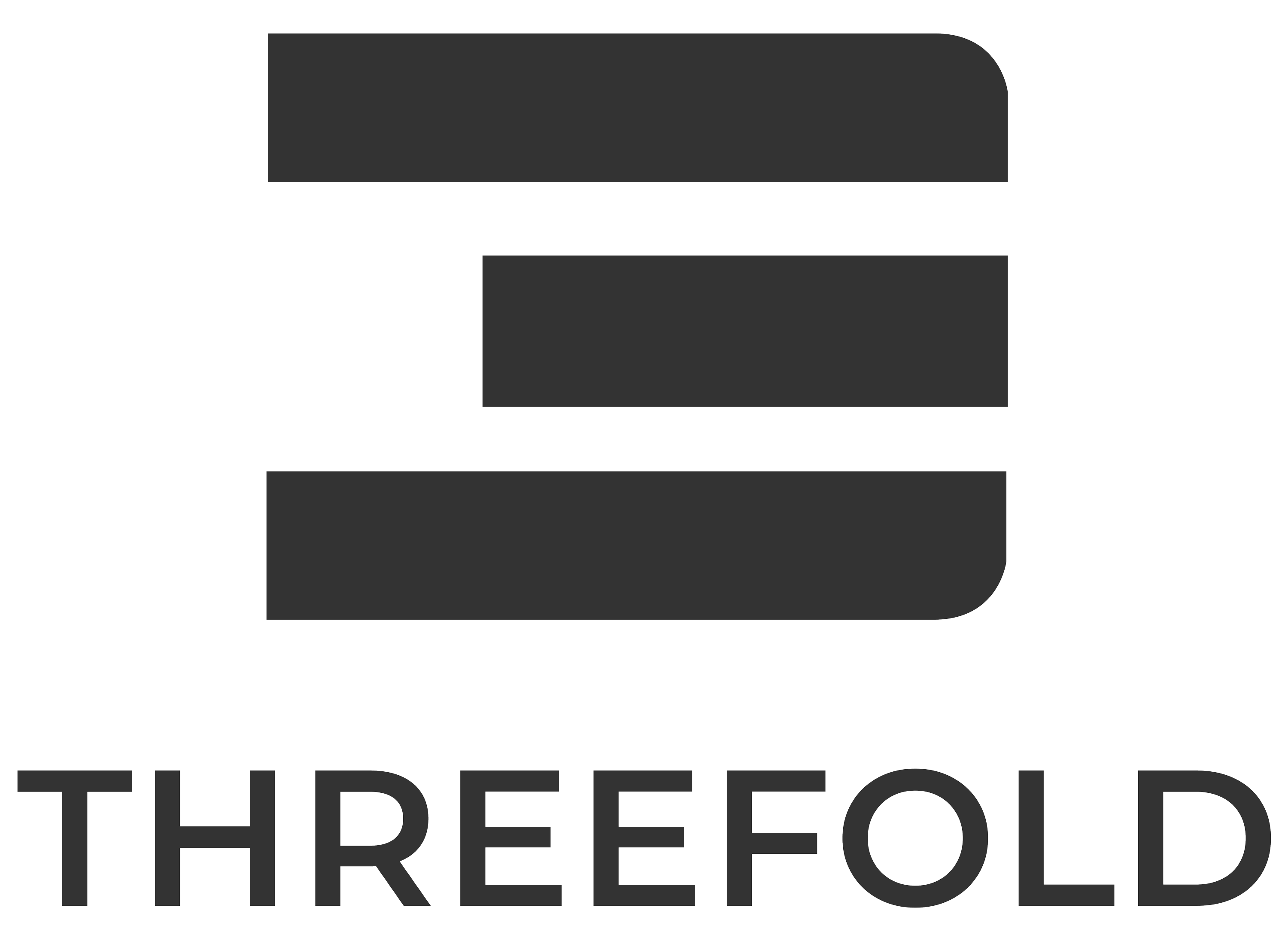
Threefold Grid v0.8.1 published on Wednesday, Nov 6, 2024 by Threefold