datarobot.RemoteRepository
Explore with Pulumi AI
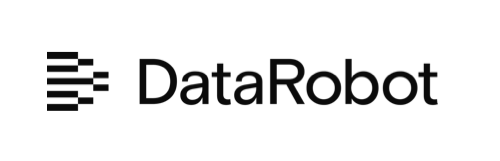
remote repository
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const githubExample = new datarobot.RemoteRepository("githubExample", {
description: "Description for the example remote repository",
location: "https://github.com/datarobot/datarobot-user-models",
sourceType: "github",
});
const gitlabExample = new datarobot.RemoteRepository("gitlabExample", {
location: "https://gitlab.yourcompany.com/username/repository",
personalAccessToken: "your_personal_access_token",
sourceType: "gitlab-cloud",
});
const bitbucketExample = new datarobot.RemoteRepository("bitbucketExample", {
location: "https://bitbucket.yourcompany.com/projects/PROJECTKEY/repos/REPONAME/browse",
sourceType: "bitbucket-server",
});
const s3Example = new datarobot.RemoteRepository("s3Example", {
location: "my-s3-bucket",
sourceType: "s3",
});
import pulumi
import pulumi_datarobot as datarobot
github_example = datarobot.RemoteRepository("githubExample",
description="Description for the example remote repository",
location="https://github.com/datarobot/datarobot-user-models",
source_type="github")
gitlab_example = datarobot.RemoteRepository("gitlabExample",
location="https://gitlab.yourcompany.com/username/repository",
personal_access_token="your_personal_access_token",
source_type="gitlab-cloud")
bitbucket_example = datarobot.RemoteRepository("bitbucketExample",
location="https://bitbucket.yourcompany.com/projects/PROJECTKEY/repos/REPONAME/browse",
source_type="bitbucket-server")
s3_example = datarobot.RemoteRepository("s3Example",
location="my-s3-bucket",
source_type="s3")
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := datarobot.NewRemoteRepository(ctx, "githubExample", &datarobot.RemoteRepositoryArgs{
Description: pulumi.String("Description for the example remote repository"),
Location: pulumi.String("https://github.com/datarobot/datarobot-user-models"),
SourceType: pulumi.String("github"),
})
if err != nil {
return err
}
_, err = datarobot.NewRemoteRepository(ctx, "gitlabExample", &datarobot.RemoteRepositoryArgs{
Location: pulumi.String("https://gitlab.yourcompany.com/username/repository"),
PersonalAccessToken: pulumi.String("your_personal_access_token"),
SourceType: pulumi.String("gitlab-cloud"),
})
if err != nil {
return err
}
_, err = datarobot.NewRemoteRepository(ctx, "bitbucketExample", &datarobot.RemoteRepositoryArgs{
Location: pulumi.String("https://bitbucket.yourcompany.com/projects/PROJECTKEY/repos/REPONAME/browse"),
SourceType: pulumi.String("bitbucket-server"),
})
if err != nil {
return err
}
_, err = datarobot.NewRemoteRepository(ctx, "s3Example", &datarobot.RemoteRepositoryArgs{
Location: pulumi.String("my-s3-bucket"),
SourceType: pulumi.String("s3"),
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var githubExample = new Datarobot.RemoteRepository("githubExample", new()
{
Description = "Description for the example remote repository",
Location = "https://github.com/datarobot/datarobot-user-models",
SourceType = "github",
});
var gitlabExample = new Datarobot.RemoteRepository("gitlabExample", new()
{
Location = "https://gitlab.yourcompany.com/username/repository",
PersonalAccessToken = "your_personal_access_token",
SourceType = "gitlab-cloud",
});
var bitbucketExample = new Datarobot.RemoteRepository("bitbucketExample", new()
{
Location = "https://bitbucket.yourcompany.com/projects/PROJECTKEY/repos/REPONAME/browse",
SourceType = "bitbucket-server",
});
var s3Example = new Datarobot.RemoteRepository("s3Example", new()
{
Location = "my-s3-bucket",
SourceType = "s3",
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.RemoteRepository;
import com.pulumi.datarobot.RemoteRepositoryArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var githubExample = new RemoteRepository("githubExample", RemoteRepositoryArgs.builder()
.description("Description for the example remote repository")
.location("https://github.com/datarobot/datarobot-user-models")
.sourceType("github")
.build());
var gitlabExample = new RemoteRepository("gitlabExample", RemoteRepositoryArgs.builder()
.location("https://gitlab.yourcompany.com/username/repository")
.personalAccessToken("your_personal_access_token")
.sourceType("gitlab-cloud")
.build());
var bitbucketExample = new RemoteRepository("bitbucketExample", RemoteRepositoryArgs.builder()
.location("https://bitbucket.yourcompany.com/projects/PROJECTKEY/repos/REPONAME/browse")
.sourceType("bitbucket-server")
.build());
var s3Example = new RemoteRepository("s3Example", RemoteRepositoryArgs.builder()
.location("my-s3-bucket")
.sourceType("s3")
.build());
}
}
resources:
githubExample:
type: datarobot:RemoteRepository
properties:
description: Description for the example remote repository
location: https://github.com/datarobot/datarobot-user-models
sourceType: github
gitlabExample:
type: datarobot:RemoteRepository
properties:
location: https://gitlab.yourcompany.com/username/repository
personalAccessToken: your_personal_access_token
sourceType: gitlab-cloud
bitbucketExample:
type: datarobot:RemoteRepository
properties:
location: https://bitbucket.yourcompany.com/projects/PROJECTKEY/repos/REPONAME/browse
sourceType: bitbucket-server
s3Example:
type: datarobot:RemoteRepository
properties:
location: my-s3-bucket
sourceType: s3
Create RemoteRepository Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new RemoteRepository(name: string, args: RemoteRepositoryArgs, opts?: CustomResourceOptions);
@overload
def RemoteRepository(resource_name: str,
args: RemoteRepositoryArgs,
opts: Optional[ResourceOptions] = None)
@overload
def RemoteRepository(resource_name: str,
opts: Optional[ResourceOptions] = None,
location: Optional[str] = None,
source_type: Optional[str] = None,
aws_access_key_id: Optional[str] = None,
aws_secret_access_key: Optional[str] = None,
aws_session_token: Optional[str] = None,
description: Optional[str] = None,
name: Optional[str] = None,
personal_access_token: Optional[str] = None)
func NewRemoteRepository(ctx *Context, name string, args RemoteRepositoryArgs, opts ...ResourceOption) (*RemoteRepository, error)
public RemoteRepository(string name, RemoteRepositoryArgs args, CustomResourceOptions? opts = null)
public RemoteRepository(String name, RemoteRepositoryArgs args)
public RemoteRepository(String name, RemoteRepositoryArgs args, CustomResourceOptions options)
type: datarobot:RemoteRepository
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args RemoteRepositoryArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args RemoteRepositoryArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args RemoteRepositoryArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args RemoteRepositoryArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args RemoteRepositoryArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var remoteRepositoryResource = new Datarobot.RemoteRepository("remoteRepositoryResource", new()
{
Location = "string",
SourceType = "string",
AwsAccessKeyId = "string",
AwsSecretAccessKey = "string",
AwsSessionToken = "string",
Description = "string",
Name = "string",
PersonalAccessToken = "string",
});
example, err := datarobot.NewRemoteRepository(ctx, "remoteRepositoryResource", &datarobot.RemoteRepositoryArgs{
Location: pulumi.String("string"),
SourceType: pulumi.String("string"),
AwsAccessKeyId: pulumi.String("string"),
AwsSecretAccessKey: pulumi.String("string"),
AwsSessionToken: pulumi.String("string"),
Description: pulumi.String("string"),
Name: pulumi.String("string"),
PersonalAccessToken: pulumi.String("string"),
})
var remoteRepositoryResource = new RemoteRepository("remoteRepositoryResource", RemoteRepositoryArgs.builder()
.location("string")
.sourceType("string")
.awsAccessKeyId("string")
.awsSecretAccessKey("string")
.awsSessionToken("string")
.description("string")
.name("string")
.personalAccessToken("string")
.build());
remote_repository_resource = datarobot.RemoteRepository("remoteRepositoryResource",
location="string",
source_type="string",
aws_access_key_id="string",
aws_secret_access_key="string",
aws_session_token="string",
description="string",
name="string",
personal_access_token="string")
const remoteRepositoryResource = new datarobot.RemoteRepository("remoteRepositoryResource", {
location: "string",
sourceType: "string",
awsAccessKeyId: "string",
awsSecretAccessKey: "string",
awsSessionToken: "string",
description: "string",
name: "string",
personalAccessToken: "string",
});
type: datarobot:RemoteRepository
properties:
awsAccessKeyId: string
awsSecretAccessKey: string
awsSessionToken: string
description: string
location: string
name: string
personalAccessToken: string
sourceType: string
RemoteRepository Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
In Python, inputs that are objects can be passed either as argument classes or as dictionary literals.
The RemoteRepository resource accepts the following input properties:
- Location string
- The location of the Remote Repository. (Bucket name for S3)
- Source
Type string - The source type of the Remote Repository.
- Aws
Access stringKey Id - The AWS access key ID for the Remote Repository.
- Aws
Secret stringAccess Key - The AWS secret access key for the Remote Repository.
- Aws
Session stringToken - The AWS session token for the Remote Repository.
- Description string
- The description of the Remote Repository.
- Name string
- The name of the Remote Repository.
- Personal
Access stringToken - The personal access token for the Remote Repository.
- Location string
- The location of the Remote Repository. (Bucket name for S3)
- Source
Type string - The source type of the Remote Repository.
- Aws
Access stringKey Id - The AWS access key ID for the Remote Repository.
- Aws
Secret stringAccess Key - The AWS secret access key for the Remote Repository.
- Aws
Session stringToken - The AWS session token for the Remote Repository.
- Description string
- The description of the Remote Repository.
- Name string
- The name of the Remote Repository.
- Personal
Access stringToken - The personal access token for the Remote Repository.
- location String
- The location of the Remote Repository. (Bucket name for S3)
- source
Type String - The source type of the Remote Repository.
- aws
Access StringKey Id - The AWS access key ID for the Remote Repository.
- aws
Secret StringAccess Key - The AWS secret access key for the Remote Repository.
- aws
Session StringToken - The AWS session token for the Remote Repository.
- description String
- The description of the Remote Repository.
- name String
- The name of the Remote Repository.
- personal
Access StringToken - The personal access token for the Remote Repository.
- location string
- The location of the Remote Repository. (Bucket name for S3)
- source
Type string - The source type of the Remote Repository.
- aws
Access stringKey Id - The AWS access key ID for the Remote Repository.
- aws
Secret stringAccess Key - The AWS secret access key for the Remote Repository.
- aws
Session stringToken - The AWS session token for the Remote Repository.
- description string
- The description of the Remote Repository.
- name string
- The name of the Remote Repository.
- personal
Access stringToken - The personal access token for the Remote Repository.
- location str
- The location of the Remote Repository. (Bucket name for S3)
- source_
type str - The source type of the Remote Repository.
- aws_
access_ strkey_ id - The AWS access key ID for the Remote Repository.
- aws_
secret_ straccess_ key - The AWS secret access key for the Remote Repository.
- aws_
session_ strtoken - The AWS session token for the Remote Repository.
- description str
- The description of the Remote Repository.
- name str
- The name of the Remote Repository.
- personal_
access_ strtoken - The personal access token for the Remote Repository.
- location String
- The location of the Remote Repository. (Bucket name for S3)
- source
Type String - The source type of the Remote Repository.
- aws
Access StringKey Id - The AWS access key ID for the Remote Repository.
- aws
Secret StringAccess Key - The AWS secret access key for the Remote Repository.
- aws
Session StringToken - The AWS session token for the Remote Repository.
- description String
- The description of the Remote Repository.
- name String
- The name of the Remote Repository.
- personal
Access StringToken - The personal access token for the Remote Repository.
Outputs
All input properties are implicitly available as output properties. Additionally, the RemoteRepository resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing RemoteRepository Resource
Get an existing RemoteRepository resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: RemoteRepositoryState, opts?: CustomResourceOptions): RemoteRepository
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
aws_access_key_id: Optional[str] = None,
aws_secret_access_key: Optional[str] = None,
aws_session_token: Optional[str] = None,
description: Optional[str] = None,
location: Optional[str] = None,
name: Optional[str] = None,
personal_access_token: Optional[str] = None,
source_type: Optional[str] = None) -> RemoteRepository
func GetRemoteRepository(ctx *Context, name string, id IDInput, state *RemoteRepositoryState, opts ...ResourceOption) (*RemoteRepository, error)
public static RemoteRepository Get(string name, Input<string> id, RemoteRepositoryState? state, CustomResourceOptions? opts = null)
public static RemoteRepository get(String name, Output<String> id, RemoteRepositoryState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Aws
Access stringKey Id - The AWS access key ID for the Remote Repository.
- Aws
Secret stringAccess Key - The AWS secret access key for the Remote Repository.
- Aws
Session stringToken - The AWS session token for the Remote Repository.
- Description string
- The description of the Remote Repository.
- Location string
- The location of the Remote Repository. (Bucket name for S3)
- Name string
- The name of the Remote Repository.
- Personal
Access stringToken - The personal access token for the Remote Repository.
- Source
Type string - The source type of the Remote Repository.
- Aws
Access stringKey Id - The AWS access key ID for the Remote Repository.
- Aws
Secret stringAccess Key - The AWS secret access key for the Remote Repository.
- Aws
Session stringToken - The AWS session token for the Remote Repository.
- Description string
- The description of the Remote Repository.
- Location string
- The location of the Remote Repository. (Bucket name for S3)
- Name string
- The name of the Remote Repository.
- Personal
Access stringToken - The personal access token for the Remote Repository.
- Source
Type string - The source type of the Remote Repository.
- aws
Access StringKey Id - The AWS access key ID for the Remote Repository.
- aws
Secret StringAccess Key - The AWS secret access key for the Remote Repository.
- aws
Session StringToken - The AWS session token for the Remote Repository.
- description String
- The description of the Remote Repository.
- location String
- The location of the Remote Repository. (Bucket name for S3)
- name String
- The name of the Remote Repository.
- personal
Access StringToken - The personal access token for the Remote Repository.
- source
Type String - The source type of the Remote Repository.
- aws
Access stringKey Id - The AWS access key ID for the Remote Repository.
- aws
Secret stringAccess Key - The AWS secret access key for the Remote Repository.
- aws
Session stringToken - The AWS session token for the Remote Repository.
- description string
- The description of the Remote Repository.
- location string
- The location of the Remote Repository. (Bucket name for S3)
- name string
- The name of the Remote Repository.
- personal
Access stringToken - The personal access token for the Remote Repository.
- source
Type string - The source type of the Remote Repository.
- aws_
access_ strkey_ id - The AWS access key ID for the Remote Repository.
- aws_
secret_ straccess_ key - The AWS secret access key for the Remote Repository.
- aws_
session_ strtoken - The AWS session token for the Remote Repository.
- description str
- The description of the Remote Repository.
- location str
- The location of the Remote Repository. (Bucket name for S3)
- name str
- The name of the Remote Repository.
- personal_
access_ strtoken - The personal access token for the Remote Repository.
- source_
type str - The source type of the Remote Repository.
- aws
Access StringKey Id - The AWS access key ID for the Remote Repository.
- aws
Secret StringAccess Key - The AWS secret access key for the Remote Repository.
- aws
Session StringToken - The AWS session token for the Remote Repository.
- description String
- The description of the Remote Repository.
- location String
- The location of the Remote Repository. (Bucket name for S3)
- name String
- The name of the Remote Repository.
- personal
Access StringToken - The personal access token for the Remote Repository.
- source
Type String - The source type of the Remote Repository.
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
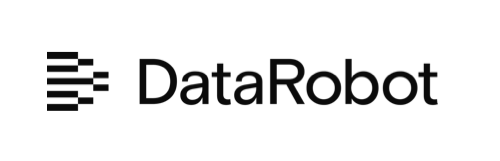