datarobot.Datastore
Explore with Pulumi AI
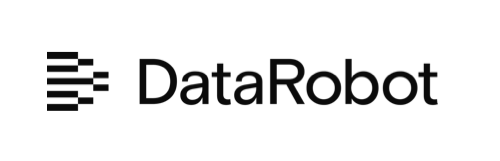
Data store
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const exampleConnector = new datarobot.Datastore("exampleConnector", {
canonicalName: "Example Connector Datastore",
dataStoreType: "dr-connector-v1",
connectorId: "65538041dde6a1d664d0b2ec",
fields: [{
id: "fs.defaultFS",
name: "Bucket Name",
value: "my-bucket",
}],
});
const exampleJdbc = new datarobot.Datastore("exampleJdbc", {
canonicalName: "Example JDBC Datastore",
dataStoreType: "jdbc",
driverId: "5b4752844bf542000175dbea",
fields: [
{
name: "address",
value: "my-address",
},
{
name: "database",
value: "my-database",
},
],
});
const exampleDatabase = new datarobot.Datastore("exampleDatabase", {
canonicalName: "Example Database Datastore",
dataStoreType: "dr-database-v1",
driverId: "64a288a50636598d75df7f82",
fields: [{
id: "bq.project_id",
name: "Project Id",
value: "project-id",
}],
});
export const exampleConnectorId = exampleConnector.id;
import pulumi
import pulumi_datarobot as datarobot
example_connector = datarobot.Datastore("exampleConnector",
canonical_name="Example Connector Datastore",
data_store_type="dr-connector-v1",
connector_id="65538041dde6a1d664d0b2ec",
fields=[{
"id": "fs.defaultFS",
"name": "Bucket Name",
"value": "my-bucket",
}])
example_jdbc = datarobot.Datastore("exampleJdbc",
canonical_name="Example JDBC Datastore",
data_store_type="jdbc",
driver_id="5b4752844bf542000175dbea",
fields=[
{
"name": "address",
"value": "my-address",
},
{
"name": "database",
"value": "my-database",
},
])
example_database = datarobot.Datastore("exampleDatabase",
canonical_name="Example Database Datastore",
data_store_type="dr-database-v1",
driver_id="64a288a50636598d75df7f82",
fields=[{
"id": "bq.project_id",
"name": "Project Id",
"value": "project-id",
}])
pulumi.export("exampleConnectorId", example_connector.id)
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
exampleConnector, err := datarobot.NewDatastore(ctx, "exampleConnector", &datarobot.DatastoreArgs{
CanonicalName: pulumi.String("Example Connector Datastore"),
DataStoreType: pulumi.String("dr-connector-v1"),
ConnectorId: pulumi.String("65538041dde6a1d664d0b2ec"),
Fields: pulumi.StringMapArray{
pulumi.StringMap{
"id": pulumi.String("fs.defaultFS"),
"name": pulumi.String("Bucket Name"),
"value": pulumi.String("my-bucket"),
},
},
})
if err != nil {
return err
}
_, err = datarobot.NewDatastore(ctx, "exampleJdbc", &datarobot.DatastoreArgs{
CanonicalName: pulumi.String("Example JDBC Datastore"),
DataStoreType: pulumi.String("jdbc"),
DriverId: pulumi.String("5b4752844bf542000175dbea"),
Fields: pulumi.StringMapArray{
pulumi.StringMap{
"name": pulumi.String("address"),
"value": pulumi.String("my-address"),
},
pulumi.StringMap{
"name": pulumi.String("database"),
"value": pulumi.String("my-database"),
},
},
})
if err != nil {
return err
}
_, err = datarobot.NewDatastore(ctx, "exampleDatabase", &datarobot.DatastoreArgs{
CanonicalName: pulumi.String("Example Database Datastore"),
DataStoreType: pulumi.String("dr-database-v1"),
DriverId: pulumi.String("64a288a50636598d75df7f82"),
Fields: pulumi.StringMapArray{
pulumi.StringMap{
"id": pulumi.String("bq.project_id"),
"name": pulumi.String("Project Id"),
"value": pulumi.String("project-id"),
},
},
})
if err != nil {
return err
}
ctx.Export("exampleConnectorId", exampleConnector.ID())
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var exampleConnector = new Datarobot.Datastore("exampleConnector", new()
{
CanonicalName = "Example Connector Datastore",
DataStoreType = "dr-connector-v1",
ConnectorId = "65538041dde6a1d664d0b2ec",
Fields = new[]
{
{
{ "id", "fs.defaultFS" },
{ "name", "Bucket Name" },
{ "value", "my-bucket" },
},
},
});
var exampleJdbc = new Datarobot.Datastore("exampleJdbc", new()
{
CanonicalName = "Example JDBC Datastore",
DataStoreType = "jdbc",
DriverId = "5b4752844bf542000175dbea",
Fields = new[]
{
{
{ "name", "address" },
{ "value", "my-address" },
},
{
{ "name", "database" },
{ "value", "my-database" },
},
},
});
var exampleDatabase = new Datarobot.Datastore("exampleDatabase", new()
{
CanonicalName = "Example Database Datastore",
DataStoreType = "dr-database-v1",
DriverId = "64a288a50636598d75df7f82",
Fields = new[]
{
{
{ "id", "bq.project_id" },
{ "name", "Project Id" },
{ "value", "project-id" },
},
},
});
return new Dictionary<string, object?>
{
["exampleConnectorId"] = exampleConnector.Id,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.Datastore;
import com.pulumi.datarobot.DatastoreArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var exampleConnector = new Datastore("exampleConnector", DatastoreArgs.builder()
.canonicalName("Example Connector Datastore")
.dataStoreType("dr-connector-v1")
.connectorId("65538041dde6a1d664d0b2ec")
.fields(Map.ofEntries(
Map.entry("id", "fs.defaultFS"),
Map.entry("name", "Bucket Name"),
Map.entry("value", "my-bucket")
))
.build());
var exampleJdbc = new Datastore("exampleJdbc", DatastoreArgs.builder()
.canonicalName("Example JDBC Datastore")
.dataStoreType("jdbc")
.driverId("5b4752844bf542000175dbea")
.fields(
Map.ofEntries(
Map.entry("name", "address"),
Map.entry("value", "my-address")
),
Map.ofEntries(
Map.entry("name", "database"),
Map.entry("value", "my-database")
))
.build());
var exampleDatabase = new Datastore("exampleDatabase", DatastoreArgs.builder()
.canonicalName("Example Database Datastore")
.dataStoreType("dr-database-v1")
.driverId("64a288a50636598d75df7f82")
.fields(Map.ofEntries(
Map.entry("id", "bq.project_id"),
Map.entry("name", "Project Id"),
Map.entry("value", "project-id")
))
.build());
ctx.export("exampleConnectorId", exampleConnector.id());
}
}
resources:
exampleConnector:
type: datarobot:Datastore
properties:
canonicalName: Example Connector Datastore
dataStoreType: dr-connector-v1
connectorId: 65538041dde6a1d664d0b2ec
fields:
- id: fs.defaultFS
name: Bucket Name
value: my-bucket
exampleJdbc:
type: datarobot:Datastore
properties:
canonicalName: Example JDBC Datastore
dataStoreType: jdbc
driverId: 5b4752844bf542000175dbea
fields:
- name: address
value: my-address
- name: database
value: my-database
exampleDatabase:
type: datarobot:Datastore
properties:
canonicalName: Example Database Datastore
dataStoreType: dr-database-v1
driverId: 64a288a50636598d75df7f82
fields:
- id: bq.project_id
name: Project Id
value: project-id
outputs:
exampleConnectorId: ${exampleConnector.id}
Create Datastore Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Datastore(name: string, args: DatastoreArgs, opts?: CustomResourceOptions);
@overload
def Datastore(resource_name: str,
args: DatastoreArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Datastore(resource_name: str,
opts: Optional[ResourceOptions] = None,
canonical_name: Optional[str] = None,
data_store_type: Optional[str] = None,
connector_id: Optional[str] = None,
driver_id: Optional[str] = None,
fields: Optional[Sequence[Mapping[str, str]]] = None,
jdbc_url: Optional[str] = None)
func NewDatastore(ctx *Context, name string, args DatastoreArgs, opts ...ResourceOption) (*Datastore, error)
public Datastore(string name, DatastoreArgs args, CustomResourceOptions? opts = null)
public Datastore(String name, DatastoreArgs args)
public Datastore(String name, DatastoreArgs args, CustomResourceOptions options)
type: datarobot:Datastore
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args DatastoreArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args DatastoreArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args DatastoreArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args DatastoreArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args DatastoreArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var datastoreResource = new Datarobot.Datastore("datastoreResource", new()
{
CanonicalName = "string",
DataStoreType = "string",
ConnectorId = "string",
DriverId = "string",
Fields = new[]
{
{
{ "string", "string" },
},
},
JdbcUrl = "string",
});
example, err := datarobot.NewDatastore(ctx, "datastoreResource", &datarobot.DatastoreArgs{
CanonicalName: pulumi.String("string"),
DataStoreType: pulumi.String("string"),
ConnectorId: pulumi.String("string"),
DriverId: pulumi.String("string"),
Fields: pulumi.StringMapArray{
pulumi.StringMap{
"string": pulumi.String("string"),
},
},
JdbcUrl: pulumi.String("string"),
})
var datastoreResource = new Datastore("datastoreResource", DatastoreArgs.builder()
.canonicalName("string")
.dataStoreType("string")
.connectorId("string")
.driverId("string")
.fields(Map.of("string", "string"))
.jdbcUrl("string")
.build());
datastore_resource = datarobot.Datastore("datastoreResource",
canonical_name="string",
data_store_type="string",
connector_id="string",
driver_id="string",
fields=[{
"string": "string",
}],
jdbc_url="string")
const datastoreResource = new datarobot.Datastore("datastoreResource", {
canonicalName: "string",
dataStoreType: "string",
connectorId: "string",
driverId: "string",
fields: [{
string: "string",
}],
jdbcUrl: "string",
});
type: datarobot:Datastore
properties:
canonicalName: string
connectorId: string
dataStoreType: string
driverId: string
fields:
- string: string
jdbcUrl: string
Datastore Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
In Python, inputs that are objects can be passed either as argument classes or as dictionary literals.
The Datastore resource accepts the following input properties:
- Canonical
Name string - The user-friendly name of the data store.
- Data
Store stringType - The type of data store.
- Connector
Id string - The identifier of the Connector if datastoretype is DRCONNECTORV1
- Driver
Id string - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- Fields
List<Immutable
Dictionary<string, string>> - If the type is dr-database-v1, then the fields specify the configuration.
- Jdbc
Url string - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- Canonical
Name string - The user-friendly name of the data store.
- Data
Store stringType - The type of data store.
- Connector
Id string - The identifier of the Connector if datastoretype is DRCONNECTORV1
- Driver
Id string - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- Fields []map[string]string
- If the type is dr-database-v1, then the fields specify the configuration.
- Jdbc
Url string - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- canonical
Name String - The user-friendly name of the data store.
- data
Store StringType - The type of data store.
- connector
Id String - The identifier of the Connector if datastoretype is DRCONNECTORV1
- driver
Id String - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- fields List<Map<String,String>>
- If the type is dr-database-v1, then the fields specify the configuration.
- jdbc
Url String - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- canonical
Name string - The user-friendly name of the data store.
- data
Store stringType - The type of data store.
- connector
Id string - The identifier of the Connector if datastoretype is DRCONNECTORV1
- driver
Id string - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- fields {[key: string]: string}[]
- If the type is dr-database-v1, then the fields specify the configuration.
- jdbc
Url string - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- canonical_
name str - The user-friendly name of the data store.
- data_
store_ strtype - The type of data store.
- connector_
id str - The identifier of the Connector if datastoretype is DRCONNECTORV1
- driver_
id str - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- fields Sequence[Mapping[str, str]]
- If the type is dr-database-v1, then the fields specify the configuration.
- jdbc_
url str - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- canonical
Name String - The user-friendly name of the data store.
- data
Store StringType - The type of data store.
- connector
Id String - The identifier of the Connector if datastoretype is DRCONNECTORV1
- driver
Id String - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- fields List<Map<String>>
- If the type is dr-database-v1, then the fields specify the configuration.
- jdbc
Url String - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
Outputs
All input properties are implicitly available as output properties. Additionally, the Datastore resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing Datastore Resource
Get an existing Datastore resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: DatastoreState, opts?: CustomResourceOptions): Datastore
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
canonical_name: Optional[str] = None,
connector_id: Optional[str] = None,
data_store_type: Optional[str] = None,
driver_id: Optional[str] = None,
fields: Optional[Sequence[Mapping[str, str]]] = None,
jdbc_url: Optional[str] = None) -> Datastore
func GetDatastore(ctx *Context, name string, id IDInput, state *DatastoreState, opts ...ResourceOption) (*Datastore, error)
public static Datastore Get(string name, Input<string> id, DatastoreState? state, CustomResourceOptions? opts = null)
public static Datastore get(String name, Output<String> id, DatastoreState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Canonical
Name string - The user-friendly name of the data store.
- Connector
Id string - The identifier of the Connector if datastoretype is DRCONNECTORV1
- Data
Store stringType - The type of data store.
- Driver
Id string - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- Fields
List<Immutable
Dictionary<string, string>> - If the type is dr-database-v1, then the fields specify the configuration.
- Jdbc
Url string - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- Canonical
Name string - The user-friendly name of the data store.
- Connector
Id string - The identifier of the Connector if datastoretype is DRCONNECTORV1
- Data
Store stringType - The type of data store.
- Driver
Id string - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- Fields []map[string]string
- If the type is dr-database-v1, then the fields specify the configuration.
- Jdbc
Url string - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- canonical
Name String - The user-friendly name of the data store.
- connector
Id String - The identifier of the Connector if datastoretype is DRCONNECTORV1
- data
Store StringType - The type of data store.
- driver
Id String - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- fields List<Map<String,String>>
- If the type is dr-database-v1, then the fields specify the configuration.
- jdbc
Url String - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- canonical
Name string - The user-friendly name of the data store.
- connector
Id string - The identifier of the Connector if datastoretype is DRCONNECTORV1
- data
Store stringType - The type of data store.
- driver
Id string - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- fields {[key: string]: string}[]
- If the type is dr-database-v1, then the fields specify the configuration.
- jdbc
Url string - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- canonical_
name str - The user-friendly name of the data store.
- connector_
id str - The identifier of the Connector if datastoretype is DRCONNECTORV1
- data_
store_ strtype - The type of data store.
- driver_
id str - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- fields Sequence[Mapping[str, str]]
- If the type is dr-database-v1, then the fields specify the configuration.
- jdbc_
url str - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
- canonical
Name String - The user-friendly name of the data store.
- connector
Id String - The identifier of the Connector if datastoretype is DRCONNECTORV1
- data
Store StringType - The type of data store.
- driver
Id String - The identifier of the DataDriver if datastoretype is JDBC or DRDATABASEV1
- fields List<Map<String>>
- If the type is dr-database-v1, then the fields specify the configuration.
- jdbc
Url String - The full JDBC URL (for example: jdbc:postgresql://my.dbaddress.org:5432/my_db).
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
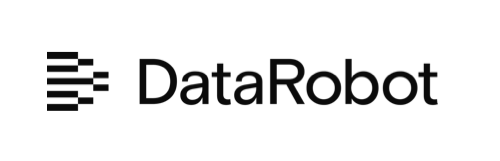