datarobot.CustomModel
Explore with Pulumi AI
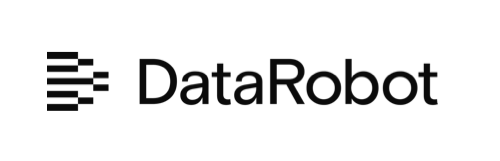
Data set from file
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const exampleRemoteRepository = new datarobot.RemoteRepository("exampleRemoteRepository", {
description: "GitHub repository with Datarobot user models",
location: "https://github.com/datarobot/datarobot-user-models",
sourceType: "github",
});
// set the credential id for private repositories
// credential_id = datarobot_api_token_credential.example.id
const exampleCustomModel = new datarobot.CustomModel("exampleCustomModel", {
description: "An example custom model from GitHub repository",
files: [
"file1.py",
"file2.py",
],
targetType: "Binary",
targetName: "my_label",
baseEnvironmentId: "65f9b27eab986d30d4c64268",
});
// Optional
// source_remote_repositories = [
// {
// id = datarobot_remote_repository.example.id
// ref = "master"
// source_paths = [
// "model_templates/python3_dummy_binary",
// ]
// }
// ]
// guard_configurations = [
// {
// template_name = "Rouge 1"
// name = "Rouge 1 response"
// stages = ["response"]
// intervention = {
// action = "block"
// message = "response has been blocked by Rogue 1 guard"
// condition = jsonencode({
// "comparand": 0.5,
// "comparator": "greaterThan"
// })
// }
// },
// ]
// overall_moderation_configuration = {
// timeout_sec = 120
// timeout_action = "score"
// }
// memory_mb = 512
// replicas = 2
// network_access = "NONE"
export const exampleId = exampleCustomModel.id;
import pulumi
import pulumi_datarobot as datarobot
example_remote_repository = datarobot.RemoteRepository("exampleRemoteRepository",
description="GitHub repository with Datarobot user models",
location="https://github.com/datarobot/datarobot-user-models",
source_type="github")
# set the credential id for private repositories
# credential_id = datarobot_api_token_credential.example.id
example_custom_model = datarobot.CustomModel("exampleCustomModel",
description="An example custom model from GitHub repository",
files=[
"file1.py",
"file2.py",
],
target_type="Binary",
target_name="my_label",
base_environment_id="65f9b27eab986d30d4c64268")
# Optional
# source_remote_repositories = [
# {
# id = datarobot_remote_repository.example.id
# ref = "master"
# source_paths = [
# "model_templates/python3_dummy_binary",
# ]
# }
# ]
# guard_configurations = [
# {
# template_name = "Rouge 1"
# name = "Rouge 1 response"
# stages = ["response"]
# intervention = {
# action = "block"
# message = "response has been blocked by Rogue 1 guard"
# condition = jsonencode({
# "comparand": 0.5,
# "comparator": "greaterThan"
# })
# }
# },
# ]
# overall_moderation_configuration = {
# timeout_sec = 120
# timeout_action = "score"
# }
# memory_mb = 512
# replicas = 2
# network_access = "NONE"
pulumi.export("exampleId", example_custom_model.id)
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := datarobot.NewRemoteRepository(ctx, "exampleRemoteRepository", &datarobot.RemoteRepositoryArgs{
Description: pulumi.String("GitHub repository with Datarobot user models"),
Location: pulumi.String("https://github.com/datarobot/datarobot-user-models"),
SourceType: pulumi.String("github"),
})
if err != nil {
return err
}
exampleCustomModel, err := datarobot.NewCustomModel(ctx, "exampleCustomModel", &datarobot.CustomModelArgs{
Description: pulumi.String("An example custom model from GitHub repository"),
Files: pulumi.Any{
"file1.py",
"file2.py",
},
TargetType: pulumi.String("Binary"),
TargetName: pulumi.String("my_label"),
BaseEnvironmentId: pulumi.String("65f9b27eab986d30d4c64268"),
})
if err != nil {
return err
}
ctx.Export("exampleId", exampleCustomModel.ID())
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var exampleRemoteRepository = new Datarobot.RemoteRepository("exampleRemoteRepository", new()
{
Description = "GitHub repository with Datarobot user models",
Location = "https://github.com/datarobot/datarobot-user-models",
SourceType = "github",
});
// set the credential id for private repositories
// credential_id = datarobot_api_token_credential.example.id
var exampleCustomModel = new Datarobot.CustomModel("exampleCustomModel", new()
{
Description = "An example custom model from GitHub repository",
Files = new[]
{
"file1.py",
"file2.py",
},
TargetType = "Binary",
TargetName = "my_label",
BaseEnvironmentId = "65f9b27eab986d30d4c64268",
});
// Optional
// source_remote_repositories = [
// {
// id = datarobot_remote_repository.example.id
// ref = "master"
// source_paths = [
// "model_templates/python3_dummy_binary",
// ]
// }
// ]
// guard_configurations = [
// {
// template_name = "Rouge 1"
// name = "Rouge 1 response"
// stages = ["response"]
// intervention = {
// action = "block"
// message = "response has been blocked by Rogue 1 guard"
// condition = jsonencode({
// "comparand": 0.5,
// "comparator": "greaterThan"
// })
// }
// },
// ]
// overall_moderation_configuration = {
// timeout_sec = 120
// timeout_action = "score"
// }
// memory_mb = 512
// replicas = 2
// network_access = "NONE"
return new Dictionary<string, object?>
{
["exampleId"] = exampleCustomModel.Id,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.RemoteRepository;
import com.pulumi.datarobot.RemoteRepositoryArgs;
import com.pulumi.datarobot.CustomModel;
import com.pulumi.datarobot.CustomModelArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var exampleRemoteRepository = new RemoteRepository("exampleRemoteRepository", RemoteRepositoryArgs.builder()
.description("GitHub repository with Datarobot user models")
.location("https://github.com/datarobot/datarobot-user-models")
.sourceType("github")
.build());
// set the credential id for private repositories
// credential_id = datarobot_api_token_credential.example.id
var exampleCustomModel = new CustomModel("exampleCustomModel", CustomModelArgs.builder()
.description("An example custom model from GitHub repository")
.files(
"file1.py",
"file2.py")
.targetType("Binary")
.targetName("my_label")
.baseEnvironmentId("65f9b27eab986d30d4c64268")
.build());
// Optional
// source_remote_repositories = [
// {
// id = datarobot_remote_repository.example.id
// ref = "master"
// source_paths = [
// "model_templates/python3_dummy_binary",
// ]
// }
// ]
// guard_configurations = [
// {
// template_name = "Rouge 1"
// name = "Rouge 1 response"
// stages = ["response"]
// intervention = {
// action = "block"
// message = "response has been blocked by Rogue 1 guard"
// condition = jsonencode({
// "comparand": 0.5,
// "comparator": "greaterThan"
// })
// }
// },
// ]
// overall_moderation_configuration = {
// timeout_sec = 120
// timeout_action = "score"
// }
// memory_mb = 512
// replicas = 2
// network_access = "NONE"
ctx.export("exampleId", exampleCustomModel.id());
}
}
resources:
exampleRemoteRepository:
type: datarobot:RemoteRepository
properties:
description: GitHub repository with Datarobot user models
location: https://github.com/datarobot/datarobot-user-models
sourceType: github
exampleCustomModel:
type: datarobot:CustomModel
properties:
description: An example custom model from GitHub repository
files:
- file1.py
- file2.py
targetType: Binary
targetName: my_label
baseEnvironmentId: 65f9b27eab986d30d4c64268
outputs:
exampleId: ${exampleCustomModel.id}
Create CustomModel Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new CustomModel(name: string, args?: CustomModelArgs, opts?: CustomResourceOptions);
@overload
def CustomModel(resource_name: str,
args: Optional[CustomModelArgs] = None,
opts: Optional[ResourceOptions] = None)
@overload
def CustomModel(resource_name: str,
opts: Optional[ResourceOptions] = None,
base_environment_id: Optional[str] = None,
base_environment_version_id: Optional[str] = None,
class_labels: Optional[Sequence[str]] = None,
class_labels_file: Optional[str] = None,
description: Optional[str] = None,
files: Optional[Any] = None,
folder_path: Optional[str] = None,
guard_configurations: Optional[Sequence[CustomModelGuardConfigurationArgs]] = None,
is_proxy: Optional[bool] = None,
language: Optional[str] = None,
memory_mb: Optional[int] = None,
name: Optional[str] = None,
negative_class_label: Optional[str] = None,
network_access: Optional[str] = None,
overall_moderation_configuration: Optional[CustomModelOverallModerationConfigurationArgs] = None,
positive_class_label: Optional[str] = None,
prediction_threshold: Optional[float] = None,
replicas: Optional[int] = None,
resource_bundle_id: Optional[str] = None,
runtime_parameter_values: Optional[Sequence[CustomModelRuntimeParameterValueArgs]] = None,
source_llm_blueprint_id: Optional[str] = None,
source_remote_repositories: Optional[Sequence[CustomModelSourceRemoteRepositoryArgs]] = None,
target_name: Optional[str] = None,
target_type: Optional[str] = None,
training_data_partition_column: Optional[str] = None,
training_dataset_id: Optional[str] = None,
use_case_ids: Optional[Sequence[str]] = None)
func NewCustomModel(ctx *Context, name string, args *CustomModelArgs, opts ...ResourceOption) (*CustomModel, error)
public CustomModel(string name, CustomModelArgs? args = null, CustomResourceOptions? opts = null)
public CustomModel(String name, CustomModelArgs args)
public CustomModel(String name, CustomModelArgs args, CustomResourceOptions options)
type: datarobot:CustomModel
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var customModelResource = new Datarobot.CustomModel("customModelResource", new()
{
BaseEnvironmentId = "string",
BaseEnvironmentVersionId = "string",
ClassLabels = new[]
{
"string",
},
ClassLabelsFile = "string",
Description = "string",
Files = "any",
FolderPath = "string",
GuardConfigurations = new[]
{
new Datarobot.Inputs.CustomModelGuardConfigurationArgs
{
Intervention = new Datarobot.Inputs.CustomModelGuardConfigurationInterventionArgs
{
Action = "string",
Condition = "string",
Message = "string",
},
Name = "string",
Stages = new[]
{
"string",
},
TemplateName = "string",
DeploymentId = "string",
InputColumnName = "string",
LlmType = "string",
NemoInfo = new Datarobot.Inputs.CustomModelGuardConfigurationNemoInfoArgs
{
Actions = "string",
BlockedTerms = "string",
LlmPrompts = "string",
MainConfig = "string",
RailsConfig = "string",
},
OpenaiApiBase = "string",
OpenaiCredential = "string",
OpenaiDeploymentId = "string",
OutputColumnName = "string",
},
},
IsProxy = false,
Language = "string",
MemoryMb = 0,
Name = "string",
NegativeClassLabel = "string",
NetworkAccess = "string",
OverallModerationConfiguration = new Datarobot.Inputs.CustomModelOverallModerationConfigurationArgs
{
TimeoutAction = "string",
TimeoutSec = 0,
},
PositiveClassLabel = "string",
PredictionThreshold = 0,
Replicas = 0,
ResourceBundleId = "string",
RuntimeParameterValues = new[]
{
new Datarobot.Inputs.CustomModelRuntimeParameterValueArgs
{
Key = "string",
Type = "string",
Value = "string",
},
},
SourceLlmBlueprintId = "string",
SourceRemoteRepositories = new[]
{
new Datarobot.Inputs.CustomModelSourceRemoteRepositoryArgs
{
Id = "string",
Ref = "string",
SourcePaths = new[]
{
"string",
},
},
},
TargetName = "string",
TargetType = "string",
TrainingDataPartitionColumn = "string",
TrainingDatasetId = "string",
UseCaseIds = new[]
{
"string",
},
});
example, err := datarobot.NewCustomModel(ctx, "customModelResource", &datarobot.CustomModelArgs{
BaseEnvironmentId: pulumi.String("string"),
BaseEnvironmentVersionId: pulumi.String("string"),
ClassLabels: pulumi.StringArray{
pulumi.String("string"),
},
ClassLabelsFile: pulumi.String("string"),
Description: pulumi.String("string"),
Files: pulumi.Any("any"),
FolderPath: pulumi.String("string"),
GuardConfigurations: datarobot.CustomModelGuardConfigurationArray{
&datarobot.CustomModelGuardConfigurationArgs{
Intervention: &datarobot.CustomModelGuardConfigurationInterventionArgs{
Action: pulumi.String("string"),
Condition: pulumi.String("string"),
Message: pulumi.String("string"),
},
Name: pulumi.String("string"),
Stages: pulumi.StringArray{
pulumi.String("string"),
},
TemplateName: pulumi.String("string"),
DeploymentId: pulumi.String("string"),
InputColumnName: pulumi.String("string"),
LlmType: pulumi.String("string"),
NemoInfo: &datarobot.CustomModelGuardConfigurationNemoInfoArgs{
Actions: pulumi.String("string"),
BlockedTerms: pulumi.String("string"),
LlmPrompts: pulumi.String("string"),
MainConfig: pulumi.String("string"),
RailsConfig: pulumi.String("string"),
},
OpenaiApiBase: pulumi.String("string"),
OpenaiCredential: pulumi.String("string"),
OpenaiDeploymentId: pulumi.String("string"),
OutputColumnName: pulumi.String("string"),
},
},
IsProxy: pulumi.Bool(false),
Language: pulumi.String("string"),
MemoryMb: pulumi.Int(0),
Name: pulumi.String("string"),
NegativeClassLabel: pulumi.String("string"),
NetworkAccess: pulumi.String("string"),
OverallModerationConfiguration: &datarobot.CustomModelOverallModerationConfigurationArgs{
TimeoutAction: pulumi.String("string"),
TimeoutSec: pulumi.Int(0),
},
PositiveClassLabel: pulumi.String("string"),
PredictionThreshold: pulumi.Float64(0),
Replicas: pulumi.Int(0),
ResourceBundleId: pulumi.String("string"),
RuntimeParameterValues: datarobot.CustomModelRuntimeParameterValueArray{
&datarobot.CustomModelRuntimeParameterValueArgs{
Key: pulumi.String("string"),
Type: pulumi.String("string"),
Value: pulumi.String("string"),
},
},
SourceLlmBlueprintId: pulumi.String("string"),
SourceRemoteRepositories: datarobot.CustomModelSourceRemoteRepositoryArray{
&datarobot.CustomModelSourceRemoteRepositoryArgs{
Id: pulumi.String("string"),
Ref: pulumi.String("string"),
SourcePaths: pulumi.StringArray{
pulumi.String("string"),
},
},
},
TargetName: pulumi.String("string"),
TargetType: pulumi.String("string"),
TrainingDataPartitionColumn: pulumi.String("string"),
TrainingDatasetId: pulumi.String("string"),
UseCaseIds: pulumi.StringArray{
pulumi.String("string"),
},
})
var customModelResource = new CustomModel("customModelResource", CustomModelArgs.builder()
.baseEnvironmentId("string")
.baseEnvironmentVersionId("string")
.classLabels("string")
.classLabelsFile("string")
.description("string")
.files("any")
.folderPath("string")
.guardConfigurations(CustomModelGuardConfigurationArgs.builder()
.intervention(CustomModelGuardConfigurationInterventionArgs.builder()
.action("string")
.condition("string")
.message("string")
.build())
.name("string")
.stages("string")
.templateName("string")
.deploymentId("string")
.inputColumnName("string")
.llmType("string")
.nemoInfo(CustomModelGuardConfigurationNemoInfoArgs.builder()
.actions("string")
.blockedTerms("string")
.llmPrompts("string")
.mainConfig("string")
.railsConfig("string")
.build())
.openaiApiBase("string")
.openaiCredential("string")
.openaiDeploymentId("string")
.outputColumnName("string")
.build())
.isProxy(false)
.language("string")
.memoryMb(0)
.name("string")
.negativeClassLabel("string")
.networkAccess("string")
.overallModerationConfiguration(CustomModelOverallModerationConfigurationArgs.builder()
.timeoutAction("string")
.timeoutSec(0)
.build())
.positiveClassLabel("string")
.predictionThreshold(0)
.replicas(0)
.resourceBundleId("string")
.runtimeParameterValues(CustomModelRuntimeParameterValueArgs.builder()
.key("string")
.type("string")
.value("string")
.build())
.sourceLlmBlueprintId("string")
.sourceRemoteRepositories(CustomModelSourceRemoteRepositoryArgs.builder()
.id("string")
.ref("string")
.sourcePaths("string")
.build())
.targetName("string")
.targetType("string")
.trainingDataPartitionColumn("string")
.trainingDatasetId("string")
.useCaseIds("string")
.build());
custom_model_resource = datarobot.CustomModel("customModelResource",
base_environment_id="string",
base_environment_version_id="string",
class_labels=["string"],
class_labels_file="string",
description="string",
files="any",
folder_path="string",
guard_configurations=[{
"intervention": {
"action": "string",
"condition": "string",
"message": "string",
},
"name": "string",
"stages": ["string"],
"template_name": "string",
"deployment_id": "string",
"input_column_name": "string",
"llm_type": "string",
"nemo_info": {
"actions": "string",
"blocked_terms": "string",
"llm_prompts": "string",
"main_config": "string",
"rails_config": "string",
},
"openai_api_base": "string",
"openai_credential": "string",
"openai_deployment_id": "string",
"output_column_name": "string",
}],
is_proxy=False,
language="string",
memory_mb=0,
name="string",
negative_class_label="string",
network_access="string",
overall_moderation_configuration={
"timeout_action": "string",
"timeout_sec": 0,
},
positive_class_label="string",
prediction_threshold=0,
replicas=0,
resource_bundle_id="string",
runtime_parameter_values=[{
"key": "string",
"type": "string",
"value": "string",
}],
source_llm_blueprint_id="string",
source_remote_repositories=[{
"id": "string",
"ref": "string",
"source_paths": ["string"],
}],
target_name="string",
target_type="string",
training_data_partition_column="string",
training_dataset_id="string",
use_case_ids=["string"])
const customModelResource = new datarobot.CustomModel("customModelResource", {
baseEnvironmentId: "string",
baseEnvironmentVersionId: "string",
classLabels: ["string"],
classLabelsFile: "string",
description: "string",
files: "any",
folderPath: "string",
guardConfigurations: [{
intervention: {
action: "string",
condition: "string",
message: "string",
},
name: "string",
stages: ["string"],
templateName: "string",
deploymentId: "string",
inputColumnName: "string",
llmType: "string",
nemoInfo: {
actions: "string",
blockedTerms: "string",
llmPrompts: "string",
mainConfig: "string",
railsConfig: "string",
},
openaiApiBase: "string",
openaiCredential: "string",
openaiDeploymentId: "string",
outputColumnName: "string",
}],
isProxy: false,
language: "string",
memoryMb: 0,
name: "string",
negativeClassLabel: "string",
networkAccess: "string",
overallModerationConfiguration: {
timeoutAction: "string",
timeoutSec: 0,
},
positiveClassLabel: "string",
predictionThreshold: 0,
replicas: 0,
resourceBundleId: "string",
runtimeParameterValues: [{
key: "string",
type: "string",
value: "string",
}],
sourceLlmBlueprintId: "string",
sourceRemoteRepositories: [{
id: "string",
ref: "string",
sourcePaths: ["string"],
}],
targetName: "string",
targetType: "string",
trainingDataPartitionColumn: "string",
trainingDatasetId: "string",
useCaseIds: ["string"],
});
type: datarobot:CustomModel
properties:
baseEnvironmentId: string
baseEnvironmentVersionId: string
classLabels:
- string
classLabelsFile: string
description: string
files: any
folderPath: string
guardConfigurations:
- deploymentId: string
inputColumnName: string
intervention:
action: string
condition: string
message: string
llmType: string
name: string
nemoInfo:
actions: string
blockedTerms: string
llmPrompts: string
mainConfig: string
railsConfig: string
openaiApiBase: string
openaiCredential: string
openaiDeploymentId: string
outputColumnName: string
stages:
- string
templateName: string
isProxy: false
language: string
memoryMb: 0
name: string
negativeClassLabel: string
networkAccess: string
overallModerationConfiguration:
timeoutAction: string
timeoutSec: 0
positiveClassLabel: string
predictionThreshold: 0
replicas: 0
resourceBundleId: string
runtimeParameterValues:
- key: string
type: string
value: string
sourceLlmBlueprintId: string
sourceRemoteRepositories:
- id: string
ref: string
sourcePaths:
- string
targetName: string
targetType: string
trainingDataPartitionColumn: string
trainingDatasetId: string
useCaseIds:
- string
CustomModel Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
In Python, inputs that are objects can be passed either as argument classes or as dictionary literals.
The CustomModel resource accepts the following input properties:
- Base
Environment stringId - The ID of the base environment for the Custom Model.
- Base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- Class
Labels List<string> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- Class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- Description string
- The description of the Custom Model.
- Files object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- Guard
Configurations List<DataRobot Custom Model Guard Configuration> - The guard configurations for the Custom Model.
- Is
Proxy bool - Flag indicating if the Custom Model is a proxy model.
- Language string
- The language used to build the Custom Model.
- Memory
Mb int - The memory in MB for the Custom Model.
- Name string
- The name of the Custom Model.
- Negative
Class stringLabel - The negative class label of the Custom Model.
- Network
Access string - The network access for the Custom Model.
- Overall
Moderation DataConfiguration Robot Custom Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- Positive
Class stringLabel - The positive class label of the Custom Model.
- Prediction
Threshold double - The prediction threshold of the Custom Model.
- Replicas int
- The replicas for the Custom Model.
- Resource
Bundle stringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- Runtime
Parameter List<DataValues Robot Custom Model Runtime Parameter Value> - The runtime parameter values for the Custom Model.
- Source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- Source
Remote List<DataRepositories Robot Custom Model Source Remote Repository> - The source remote repositories for the Custom Model.
- Target
Name string - The target name of the Custom Model.
- Target
Type string - The target type of the Custom Model.
- Training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- Training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- Use
Case List<string>Ids - The list of Use Case IDs to add the Custom Model version to.
- Base
Environment stringId - The ID of the base environment for the Custom Model.
- Base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- Class
Labels []string - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- Class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- Description string
- The description of the Custom Model.
- Files interface{}
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- Guard
Configurations []CustomModel Guard Configuration Args - The guard configurations for the Custom Model.
- Is
Proxy bool - Flag indicating if the Custom Model is a proxy model.
- Language string
- The language used to build the Custom Model.
- Memory
Mb int - The memory in MB for the Custom Model.
- Name string
- The name of the Custom Model.
- Negative
Class stringLabel - The negative class label of the Custom Model.
- Network
Access string - The network access for the Custom Model.
- Overall
Moderation CustomConfiguration Model Overall Moderation Configuration Args - The overall moderation configuration for the Custom Model.
- Positive
Class stringLabel - The positive class label of the Custom Model.
- Prediction
Threshold float64 - The prediction threshold of the Custom Model.
- Replicas int
- The replicas for the Custom Model.
- Resource
Bundle stringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- Runtime
Parameter []CustomValues Model Runtime Parameter Value Args - The runtime parameter values for the Custom Model.
- Source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- Source
Remote []CustomRepositories Model Source Remote Repository Args - The source remote repositories for the Custom Model.
- Target
Name string - The target name of the Custom Model.
- Target
Type string - The target type of the Custom Model.
- Training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- Training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- Use
Case []stringIds - The list of Use Case IDs to add the Custom Model version to.
- base
Environment StringId - The ID of the base environment for the Custom Model.
- base
Environment StringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels List<String> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels StringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- description String
- The description of the Custom Model.
- files Object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard
Configurations List<CustomModel Guard Configuration> - The guard configurations for the Custom Model.
- is
Proxy Boolean - Flag indicating if the Custom Model is a proxy model.
- language String
- The language used to build the Custom Model.
- memory
Mb Integer - The memory in MB for the Custom Model.
- name String
- The name of the Custom Model.
- negative
Class StringLabel - The negative class label of the Custom Model.
- network
Access String - The network access for the Custom Model.
- overall
Moderation CustomConfiguration Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- positive
Class StringLabel - The positive class label of the Custom Model.
- prediction
Threshold Double - The prediction threshold of the Custom Model.
- replicas Integer
- The replicas for the Custom Model.
- resource
Bundle StringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- runtime
Parameter List<CustomValues Model Runtime Parameter Value> - The runtime parameter values for the Custom Model.
- source
Llm StringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote List<CustomRepositories Model Source Remote Repository> - The source remote repositories for the Custom Model.
- target
Name String - The target name of the Custom Model.
- target
Type String - The target type of the Custom Model.
- training
Data StringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset StringId - The ID of the training dataset assigned to the Custom Model.
- use
Case List<String>Ids - The list of Use Case IDs to add the Custom Model version to.
- base
Environment stringId - The ID of the base environment for the Custom Model.
- base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels string[] - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- description string
- The description of the Custom Model.
- files any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard
Configurations CustomModel Guard Configuration[] - The guard configurations for the Custom Model.
- is
Proxy boolean - Flag indicating if the Custom Model is a proxy model.
- language string
- The language used to build the Custom Model.
- memory
Mb number - The memory in MB for the Custom Model.
- name string
- The name of the Custom Model.
- negative
Class stringLabel - The negative class label of the Custom Model.
- network
Access string - The network access for the Custom Model.
- overall
Moderation CustomConfiguration Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- positive
Class stringLabel - The positive class label of the Custom Model.
- prediction
Threshold number - The prediction threshold of the Custom Model.
- replicas number
- The replicas for the Custom Model.
- resource
Bundle stringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- runtime
Parameter CustomValues Model Runtime Parameter Value[] - The runtime parameter values for the Custom Model.
- source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote CustomRepositories Model Source Remote Repository[] - The source remote repositories for the Custom Model.
- target
Name string - The target name of the Custom Model.
- target
Type string - The target type of the Custom Model.
- training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- use
Case string[]Ids - The list of Use Case IDs to add the Custom Model version to.
- base_
environment_ strid - The ID of the base environment for the Custom Model.
- base_
environment_ strversion_ id - The ID of the base environment version for the Custom Model.
- class_
labels Sequence[str] - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class_
labels_ strfile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- description str
- The description of the Custom Model.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder_
path str - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard_
configurations Sequence[CustomModel Guard Configuration Args] - The guard configurations for the Custom Model.
- is_
proxy bool - Flag indicating if the Custom Model is a proxy model.
- language str
- The language used to build the Custom Model.
- memory_
mb int - The memory in MB for the Custom Model.
- name str
- The name of the Custom Model.
- negative_
class_ strlabel - The negative class label of the Custom Model.
- network_
access str - The network access for the Custom Model.
- overall_
moderation_ Customconfiguration Model Overall Moderation Configuration Args - The overall moderation configuration for the Custom Model.
- positive_
class_ strlabel - The positive class label of the Custom Model.
- prediction_
threshold float - The prediction threshold of the Custom Model.
- replicas int
- The replicas for the Custom Model.
- resource_
bundle_ strid - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- runtime_
parameter_ Sequence[Customvalues Model Runtime Parameter Value Args] - The runtime parameter values for the Custom Model.
- source_
llm_ strblueprint_ id - The ID of the source LLM Blueprint for the Custom Model.
- source_
remote_ Sequence[Customrepositories Model Source Remote Repository Args] - The source remote repositories for the Custom Model.
- target_
name str - The target name of the Custom Model.
- target_
type str - The target type of the Custom Model.
- training_
data_ strpartition_ column - The name of the partition column in the training dataset assigned to the Custom Model.
- training_
dataset_ strid - The ID of the training dataset assigned to the Custom Model.
- use_
case_ Sequence[str]ids - The list of Use Case IDs to add the Custom Model version to.
- base
Environment StringId - The ID of the base environment for the Custom Model.
- base
Environment StringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels List<String> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels StringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- description String
- The description of the Custom Model.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard
Configurations List<Property Map> - The guard configurations for the Custom Model.
- is
Proxy Boolean - Flag indicating if the Custom Model is a proxy model.
- language String
- The language used to build the Custom Model.
- memory
Mb Number - The memory in MB for the Custom Model.
- name String
- The name of the Custom Model.
- negative
Class StringLabel - The negative class label of the Custom Model.
- network
Access String - The network access for the Custom Model.
- overall
Moderation Property MapConfiguration - The overall moderation configuration for the Custom Model.
- positive
Class StringLabel - The positive class label of the Custom Model.
- prediction
Threshold Number - The prediction threshold of the Custom Model.
- replicas Number
- The replicas for the Custom Model.
- resource
Bundle StringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- runtime
Parameter List<Property Map>Values - The runtime parameter values for the Custom Model.
- source
Llm StringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote List<Property Map>Repositories - The source remote repositories for the Custom Model.
- target
Name String - The target name of the Custom Model.
- target
Type String - The target type of the Custom Model.
- training
Data StringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset StringId - The ID of the training dataset assigned to the Custom Model.
- use
Case List<String>Ids - The list of Use Case IDs to add the Custom Model version to.
Outputs
All input properties are implicitly available as output properties. Additionally, the CustomModel resource produces the following output properties:
- Deployments
Count int - The number of deployments for the Custom Model.
- Files
Hashes List<string> - The hash of file contents for each file in files.
- Folder
Path stringHash - The hash of the folder path contents.
- Id string
- The provider-assigned unique ID for this managed resource.
- Training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- Training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- Version
Id string - The ID of the latest Custom Model version.
- Deployments
Count int - The number of deployments for the Custom Model.
- Files
Hashes []string - The hash of file contents for each file in files.
- Folder
Path stringHash - The hash of the folder path contents.
- Id string
- The provider-assigned unique ID for this managed resource.
- Training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- Training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- Version
Id string - The ID of the latest Custom Model version.
- deployments
Count Integer - The number of deployments for the Custom Model.
- files
Hashes List<String> - The hash of file contents for each file in files.
- folder
Path StringHash - The hash of the folder path contents.
- id String
- The provider-assigned unique ID for this managed resource.
- training
Dataset StringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset StringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- version
Id String - The ID of the latest Custom Model version.
- deployments
Count number - The number of deployments for the Custom Model.
- files
Hashes string[] - The hash of file contents for each file in files.
- folder
Path stringHash - The hash of the folder path contents.
- id string
- The provider-assigned unique ID for this managed resource.
- training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- version
Id string - The ID of the latest Custom Model version.
- deployments_
count int - The number of deployments for the Custom Model.
- files_
hashes Sequence[str] - The hash of file contents for each file in files.
- folder_
path_ strhash - The hash of the folder path contents.
- id str
- The provider-assigned unique ID for this managed resource.
- training_
dataset_ strname - The name of the training dataset assigned to the Custom Model.
- training_
dataset_ strversion_ id - The version ID of the training dataset assigned to the Custom Model.
- version_
id str - The ID of the latest Custom Model version.
- deployments
Count Number - The number of deployments for the Custom Model.
- files
Hashes List<String> - The hash of file contents for each file in files.
- folder
Path StringHash - The hash of the folder path contents.
- id String
- The provider-assigned unique ID for this managed resource.
- training
Dataset StringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset StringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- version
Id String - The ID of the latest Custom Model version.
Look up Existing CustomModel Resource
Get an existing CustomModel resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: CustomModelState, opts?: CustomResourceOptions): CustomModel
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
base_environment_id: Optional[str] = None,
base_environment_version_id: Optional[str] = None,
class_labels: Optional[Sequence[str]] = None,
class_labels_file: Optional[str] = None,
deployments_count: Optional[int] = None,
description: Optional[str] = None,
files: Optional[Any] = None,
files_hashes: Optional[Sequence[str]] = None,
folder_path: Optional[str] = None,
folder_path_hash: Optional[str] = None,
guard_configurations: Optional[Sequence[CustomModelGuardConfigurationArgs]] = None,
is_proxy: Optional[bool] = None,
language: Optional[str] = None,
memory_mb: Optional[int] = None,
name: Optional[str] = None,
negative_class_label: Optional[str] = None,
network_access: Optional[str] = None,
overall_moderation_configuration: Optional[CustomModelOverallModerationConfigurationArgs] = None,
positive_class_label: Optional[str] = None,
prediction_threshold: Optional[float] = None,
replicas: Optional[int] = None,
resource_bundle_id: Optional[str] = None,
runtime_parameter_values: Optional[Sequence[CustomModelRuntimeParameterValueArgs]] = None,
source_llm_blueprint_id: Optional[str] = None,
source_remote_repositories: Optional[Sequence[CustomModelSourceRemoteRepositoryArgs]] = None,
target_name: Optional[str] = None,
target_type: Optional[str] = None,
training_data_partition_column: Optional[str] = None,
training_dataset_id: Optional[str] = None,
training_dataset_name: Optional[str] = None,
training_dataset_version_id: Optional[str] = None,
use_case_ids: Optional[Sequence[str]] = None,
version_id: Optional[str] = None) -> CustomModel
func GetCustomModel(ctx *Context, name string, id IDInput, state *CustomModelState, opts ...ResourceOption) (*CustomModel, error)
public static CustomModel Get(string name, Input<string> id, CustomModelState? state, CustomResourceOptions? opts = null)
public static CustomModel get(String name, Output<String> id, CustomModelState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Base
Environment stringId - The ID of the base environment for the Custom Model.
- Base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- Class
Labels List<string> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- Class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- Deployments
Count int - The number of deployments for the Custom Model.
- Description string
- The description of the Custom Model.
- Files object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- Files
Hashes List<string> - The hash of file contents for each file in files.
- Folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- Folder
Path stringHash - The hash of the folder path contents.
- Guard
Configurations List<DataRobot Custom Model Guard Configuration> - The guard configurations for the Custom Model.
- Is
Proxy bool - Flag indicating if the Custom Model is a proxy model.
- Language string
- The language used to build the Custom Model.
- Memory
Mb int - The memory in MB for the Custom Model.
- Name string
- The name of the Custom Model.
- Negative
Class stringLabel - The negative class label of the Custom Model.
- Network
Access string - The network access for the Custom Model.
- Overall
Moderation DataConfiguration Robot Custom Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- Positive
Class stringLabel - The positive class label of the Custom Model.
- Prediction
Threshold double - The prediction threshold of the Custom Model.
- Replicas int
- The replicas for the Custom Model.
- Resource
Bundle stringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- Runtime
Parameter List<DataValues Robot Custom Model Runtime Parameter Value> - The runtime parameter values for the Custom Model.
- Source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- Source
Remote List<DataRepositories Robot Custom Model Source Remote Repository> - The source remote repositories for the Custom Model.
- Target
Name string - The target name of the Custom Model.
- Target
Type string - The target type of the Custom Model.
- Training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- Training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- Training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- Training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- Use
Case List<string>Ids - The list of Use Case IDs to add the Custom Model version to.
- Version
Id string - The ID of the latest Custom Model version.
- Base
Environment stringId - The ID of the base environment for the Custom Model.
- Base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- Class
Labels []string - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- Class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- Deployments
Count int - The number of deployments for the Custom Model.
- Description string
- The description of the Custom Model.
- Files interface{}
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- Files
Hashes []string - The hash of file contents for each file in files.
- Folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- Folder
Path stringHash - The hash of the folder path contents.
- Guard
Configurations []CustomModel Guard Configuration Args - The guard configurations for the Custom Model.
- Is
Proxy bool - Flag indicating if the Custom Model is a proxy model.
- Language string
- The language used to build the Custom Model.
- Memory
Mb int - The memory in MB for the Custom Model.
- Name string
- The name of the Custom Model.
- Negative
Class stringLabel - The negative class label of the Custom Model.
- Network
Access string - The network access for the Custom Model.
- Overall
Moderation CustomConfiguration Model Overall Moderation Configuration Args - The overall moderation configuration for the Custom Model.
- Positive
Class stringLabel - The positive class label of the Custom Model.
- Prediction
Threshold float64 - The prediction threshold of the Custom Model.
- Replicas int
- The replicas for the Custom Model.
- Resource
Bundle stringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- Runtime
Parameter []CustomValues Model Runtime Parameter Value Args - The runtime parameter values for the Custom Model.
- Source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- Source
Remote []CustomRepositories Model Source Remote Repository Args - The source remote repositories for the Custom Model.
- Target
Name string - The target name of the Custom Model.
- Target
Type string - The target type of the Custom Model.
- Training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- Training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- Training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- Training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- Use
Case []stringIds - The list of Use Case IDs to add the Custom Model version to.
- Version
Id string - The ID of the latest Custom Model version.
- base
Environment StringId - The ID of the base environment for the Custom Model.
- base
Environment StringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels List<String> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels StringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- deployments
Count Integer - The number of deployments for the Custom Model.
- description String
- The description of the Custom Model.
- files Object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- files
Hashes List<String> - The hash of file contents for each file in files.
- folder
Path String - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- folder
Path StringHash - The hash of the folder path contents.
- guard
Configurations List<CustomModel Guard Configuration> - The guard configurations for the Custom Model.
- is
Proxy Boolean - Flag indicating if the Custom Model is a proxy model.
- language String
- The language used to build the Custom Model.
- memory
Mb Integer - The memory in MB for the Custom Model.
- name String
- The name of the Custom Model.
- negative
Class StringLabel - The negative class label of the Custom Model.
- network
Access String - The network access for the Custom Model.
- overall
Moderation CustomConfiguration Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- positive
Class StringLabel - The positive class label of the Custom Model.
- prediction
Threshold Double - The prediction threshold of the Custom Model.
- replicas Integer
- The replicas for the Custom Model.
- resource
Bundle StringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- runtime
Parameter List<CustomValues Model Runtime Parameter Value> - The runtime parameter values for the Custom Model.
- source
Llm StringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote List<CustomRepositories Model Source Remote Repository> - The source remote repositories for the Custom Model.
- target
Name String - The target name of the Custom Model.
- target
Type String - The target type of the Custom Model.
- training
Data StringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset StringId - The ID of the training dataset assigned to the Custom Model.
- training
Dataset StringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset StringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- use
Case List<String>Ids - The list of Use Case IDs to add the Custom Model version to.
- version
Id String - The ID of the latest Custom Model version.
- base
Environment stringId - The ID of the base environment for the Custom Model.
- base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels string[] - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- deployments
Count number - The number of deployments for the Custom Model.
- description string
- The description of the Custom Model.
- files any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- files
Hashes string[] - The hash of file contents for each file in files.
- folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- folder
Path stringHash - The hash of the folder path contents.
- guard
Configurations CustomModel Guard Configuration[] - The guard configurations for the Custom Model.
- is
Proxy boolean - Flag indicating if the Custom Model is a proxy model.
- language string
- The language used to build the Custom Model.
- memory
Mb number - The memory in MB for the Custom Model.
- name string
- The name of the Custom Model.
- negative
Class stringLabel - The negative class label of the Custom Model.
- network
Access string - The network access for the Custom Model.
- overall
Moderation CustomConfiguration Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- positive
Class stringLabel - The positive class label of the Custom Model.
- prediction
Threshold number - The prediction threshold of the Custom Model.
- replicas number
- The replicas for the Custom Model.
- resource
Bundle stringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- runtime
Parameter CustomValues Model Runtime Parameter Value[] - The runtime parameter values for the Custom Model.
- source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote CustomRepositories Model Source Remote Repository[] - The source remote repositories for the Custom Model.
- target
Name string - The target name of the Custom Model.
- target
Type string - The target type of the Custom Model.
- training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- use
Case string[]Ids - The list of Use Case IDs to add the Custom Model version to.
- version
Id string - The ID of the latest Custom Model version.
- base_
environment_ strid - The ID of the base environment for the Custom Model.
- base_
environment_ strversion_ id - The ID of the base environment version for the Custom Model.
- class_
labels Sequence[str] - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class_
labels_ strfile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- deployments_
count int - The number of deployments for the Custom Model.
- description str
- The description of the Custom Model.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- files_
hashes Sequence[str] - The hash of file contents for each file in files.
- folder_
path str - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- folder_
path_ strhash - The hash of the folder path contents.
- guard_
configurations Sequence[CustomModel Guard Configuration Args] - The guard configurations for the Custom Model.
- is_
proxy bool - Flag indicating if the Custom Model is a proxy model.
- language str
- The language used to build the Custom Model.
- memory_
mb int - The memory in MB for the Custom Model.
- name str
- The name of the Custom Model.
- negative_
class_ strlabel - The negative class label of the Custom Model.
- network_
access str - The network access for the Custom Model.
- overall_
moderation_ Customconfiguration Model Overall Moderation Configuration Args - The overall moderation configuration for the Custom Model.
- positive_
class_ strlabel - The positive class label of the Custom Model.
- prediction_
threshold float - The prediction threshold of the Custom Model.
- replicas int
- The replicas for the Custom Model.
- resource_
bundle_ strid - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- runtime_
parameter_ Sequence[Customvalues Model Runtime Parameter Value Args] - The runtime parameter values for the Custom Model.
- source_
llm_ strblueprint_ id - The ID of the source LLM Blueprint for the Custom Model.
- source_
remote_ Sequence[Customrepositories Model Source Remote Repository Args] - The source remote repositories for the Custom Model.
- target_
name str - The target name of the Custom Model.
- target_
type str - The target type of the Custom Model.
- training_
data_ strpartition_ column - The name of the partition column in the training dataset assigned to the Custom Model.
- training_
dataset_ strid - The ID of the training dataset assigned to the Custom Model.
- training_
dataset_ strname - The name of the training dataset assigned to the Custom Model.
- training_
dataset_ strversion_ id - The version ID of the training dataset assigned to the Custom Model.
- use_
case_ Sequence[str]ids - The list of Use Case IDs to add the Custom Model version to.
- version_
id str - The ID of the latest Custom Model version.
- base
Environment StringId - The ID of the base environment for the Custom Model.
- base
Environment StringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels List<String> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels StringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- deployments
Count Number - The number of deployments for the Custom Model.
- description String
- The description of the Custom Model.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- files
Hashes List<String> - The hash of file contents for each file in files.
- folder
Path String - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- folder
Path StringHash - The hash of the folder path contents.
- guard
Configurations List<Property Map> - The guard configurations for the Custom Model.
- is
Proxy Boolean - Flag indicating if the Custom Model is a proxy model.
- language String
- The language used to build the Custom Model.
- memory
Mb Number - The memory in MB for the Custom Model.
- name String
- The name of the Custom Model.
- negative
Class StringLabel - The negative class label of the Custom Model.
- network
Access String - The network access for the Custom Model.
- overall
Moderation Property MapConfiguration - The overall moderation configuration for the Custom Model.
- positive
Class StringLabel - The positive class label of the Custom Model.
- prediction
Threshold Number - The prediction threshold of the Custom Model.
- replicas Number
- The replicas for the Custom Model.
- resource
Bundle StringId - A single identifier that represents a bundle of resources: Memory, CPU, GPU, etc.
- runtime
Parameter List<Property Map>Values - The runtime parameter values for the Custom Model.
- source
Llm StringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote List<Property Map>Repositories - The source remote repositories for the Custom Model.
- target
Name String - The target name of the Custom Model.
- target
Type String - The target type of the Custom Model.
- training
Data StringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset StringId - The ID of the training dataset assigned to the Custom Model.
- training
Dataset StringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset StringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- use
Case List<String>Ids - The list of Use Case IDs to add the Custom Model version to.
- version
Id String - The ID of the latest Custom Model version.
Supporting Types
CustomModelGuardConfiguration, CustomModelGuardConfigurationArgs
- Intervention
Data
Robot Custom Model Guard Configuration Intervention - The intervention for the guard configuration.
- Name string
- The name of the guard configuration.
- Stages List<string>
- The list of stages for the guard configuration.
- Template
Name string - The template name of the guard configuration.
- Deployment
Id string - The deployment ID of this guard.
- Input
Column stringName - The input column name of this guard.
- Llm
Type string - The LLM type for this guard.
- Nemo
Info DataRobot Custom Model Guard Configuration Nemo Info - Configuration info for NeMo guards.
- Openai
Api stringBase - The OpenAI API base URL for this guard.
- Openai
Credential string - The ID of an OpenAI credential for this guard.
- Openai
Deployment stringId - The ID of an OpenAI deployment for this guard.
- Output
Column stringName - The output column name of this guard.
- Intervention
Custom
Model Guard Configuration Intervention - The intervention for the guard configuration.
- Name string
- The name of the guard configuration.
- Stages []string
- The list of stages for the guard configuration.
- Template
Name string - The template name of the guard configuration.
- Deployment
Id string - The deployment ID of this guard.
- Input
Column stringName - The input column name of this guard.
- Llm
Type string - The LLM type for this guard.
- Nemo
Info CustomModel Guard Configuration Nemo Info - Configuration info for NeMo guards.
- Openai
Api stringBase - The OpenAI API base URL for this guard.
- Openai
Credential string - The ID of an OpenAI credential for this guard.
- Openai
Deployment stringId - The ID of an OpenAI deployment for this guard.
- Output
Column stringName - The output column name of this guard.
- intervention
Custom
Model Guard Configuration Intervention - The intervention for the guard configuration.
- name String
- The name of the guard configuration.
- stages List<String>
- The list of stages for the guard configuration.
- template
Name String - The template name of the guard configuration.
- deployment
Id String - The deployment ID of this guard.
- input
Column StringName - The input column name of this guard.
- llm
Type String - The LLM type for this guard.
- nemo
Info CustomModel Guard Configuration Nemo Info - Configuration info for NeMo guards.
- openai
Api StringBase - The OpenAI API base URL for this guard.
- openai
Credential String - The ID of an OpenAI credential for this guard.
- openai
Deployment StringId - The ID of an OpenAI deployment for this guard.
- output
Column StringName - The output column name of this guard.
- intervention
Custom
Model Guard Configuration Intervention - The intervention for the guard configuration.
- name string
- The name of the guard configuration.
- stages string[]
- The list of stages for the guard configuration.
- template
Name string - The template name of the guard configuration.
- deployment
Id string - The deployment ID of this guard.
- input
Column stringName - The input column name of this guard.
- llm
Type string - The LLM type for this guard.
- nemo
Info CustomModel Guard Configuration Nemo Info - Configuration info for NeMo guards.
- openai
Api stringBase - The OpenAI API base URL for this guard.
- openai
Credential string - The ID of an OpenAI credential for this guard.
- openai
Deployment stringId - The ID of an OpenAI deployment for this guard.
- output
Column stringName - The output column name of this guard.
- intervention
Custom
Model Guard Configuration Intervention - The intervention for the guard configuration.
- name str
- The name of the guard configuration.
- stages Sequence[str]
- The list of stages for the guard configuration.
- template_
name str - The template name of the guard configuration.
- deployment_
id str - The deployment ID of this guard.
- input_
column_ strname - The input column name of this guard.
- llm_
type str - The LLM type for this guard.
- nemo_
info CustomModel Guard Configuration Nemo Info - Configuration info for NeMo guards.
- openai_
api_ strbase - The OpenAI API base URL for this guard.
- openai_
credential str - The ID of an OpenAI credential for this guard.
- openai_
deployment_ strid - The ID of an OpenAI deployment for this guard.
- output_
column_ strname - The output column name of this guard.
- intervention Property Map
- The intervention for the guard configuration.
- name String
- The name of the guard configuration.
- stages List<String>
- The list of stages for the guard configuration.
- template
Name String - The template name of the guard configuration.
- deployment
Id String - The deployment ID of this guard.
- input
Column StringName - The input column name of this guard.
- llm
Type String - The LLM type for this guard.
- nemo
Info Property Map - Configuration info for NeMo guards.
- openai
Api StringBase - The OpenAI API base URL for this guard.
- openai
Credential String - The ID of an OpenAI credential for this guard.
- openai
Deployment StringId - The ID of an OpenAI deployment for this guard.
- output
Column StringName - The output column name of this guard.
CustomModelGuardConfigurationIntervention, CustomModelGuardConfigurationInterventionArgs
CustomModelGuardConfigurationNemoInfo, CustomModelGuardConfigurationNemoInfoArgs
- Actions string
- The actions for the NeMo information.
- Blocked
Terms string - NeMo guardrails blocked terms list.
- Llm
Prompts string - NeMo guardrails prompts.
- Main
Config string - Overall NeMo configuration YAML.
- Rails
Config string - NeMo guardrails configuration Colang.
- Actions string
- The actions for the NeMo information.
- Blocked
Terms string - NeMo guardrails blocked terms list.
- Llm
Prompts string - NeMo guardrails prompts.
- Main
Config string - Overall NeMo configuration YAML.
- Rails
Config string - NeMo guardrails configuration Colang.
- actions String
- The actions for the NeMo information.
- blocked
Terms String - NeMo guardrails blocked terms list.
- llm
Prompts String - NeMo guardrails prompts.
- main
Config String - Overall NeMo configuration YAML.
- rails
Config String - NeMo guardrails configuration Colang.
- actions string
- The actions for the NeMo information.
- blocked
Terms string - NeMo guardrails blocked terms list.
- llm
Prompts string - NeMo guardrails prompts.
- main
Config string - Overall NeMo configuration YAML.
- rails
Config string - NeMo guardrails configuration Colang.
- actions str
- The actions for the NeMo information.
- blocked_
terms str - NeMo guardrails blocked terms list.
- llm_
prompts str - NeMo guardrails prompts.
- main_
config str - Overall NeMo configuration YAML.
- rails_
config str - NeMo guardrails configuration Colang.
- actions String
- The actions for the NeMo information.
- blocked
Terms String - NeMo guardrails blocked terms list.
- llm
Prompts String - NeMo guardrails prompts.
- main
Config String - Overall NeMo configuration YAML.
- rails
Config String - NeMo guardrails configuration Colang.
CustomModelOverallModerationConfiguration, CustomModelOverallModerationConfigurationArgs
- Timeout
Action string - The timeout action of the overall moderation configuration.
- Timeout
Sec int - The timeout in seconds of the overall moderation configuration.
- Timeout
Action string - The timeout action of the overall moderation configuration.
- Timeout
Sec int - The timeout in seconds of the overall moderation configuration.
- timeout
Action String - The timeout action of the overall moderation configuration.
- timeout
Sec Integer - The timeout in seconds of the overall moderation configuration.
- timeout
Action string - The timeout action of the overall moderation configuration.
- timeout
Sec number - The timeout in seconds of the overall moderation configuration.
- timeout_
action str - The timeout action of the overall moderation configuration.
- timeout_
sec int - The timeout in seconds of the overall moderation configuration.
- timeout
Action String - The timeout action of the overall moderation configuration.
- timeout
Sec Number - The timeout in seconds of the overall moderation configuration.
CustomModelRuntimeParameterValue, CustomModelRuntimeParameterValueArgs
CustomModelSourceRemoteRepository, CustomModelSourceRemoteRepositoryArgs
- Id string
- The ID of the source remote repository.
- Ref string
- The reference of the source remote repository.
- Source
Paths List<string> - The list of source paths in the source remote repository.
- Id string
- The ID of the source remote repository.
- Ref string
- The reference of the source remote repository.
- Source
Paths []string - The list of source paths in the source remote repository.
- id String
- The ID of the source remote repository.
- ref String
- The reference of the source remote repository.
- source
Paths List<String> - The list of source paths in the source remote repository.
- id string
- The ID of the source remote repository.
- ref string
- The reference of the source remote repository.
- source
Paths string[] - The list of source paths in the source remote repository.
- id str
- The ID of the source remote repository.
- ref str
- The reference of the source remote repository.
- source_
paths Sequence[str] - The list of source paths in the source remote repository.
- id String
- The ID of the source remote repository.
- ref String
- The reference of the source remote repository.
- source
Paths List<String> - The list of source paths in the source remote repository.
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
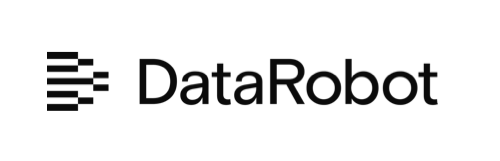